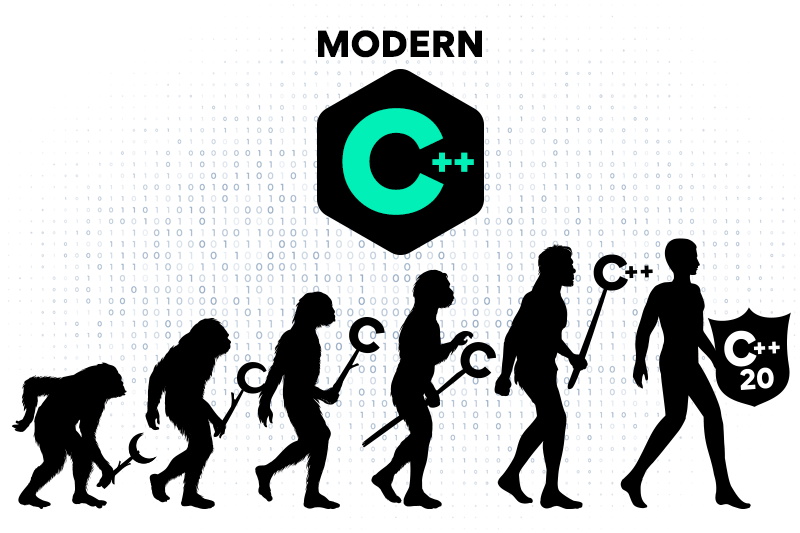
Evolution of C++ Programming Language
History and Background of C++
The C++ programming language has a captivating history that traces back to the early 1980s when Bjarne Stroustrup, a Danish computer scientist, set out to add object-oriented features to the C language. This endeavor began at Bell Labs, where he wanted to enhance programming for system software while maintaining the efficiency of C.
From its inception, C++ was molded around core principles such as:
- Object-Oriented Programming (OOP): Allowing for easier data management and reuse of code.
- Low-Level manipulation: Providing programmers the ability to work closely with hardware when necessary.
Over the years, the language saw several updates, with C++98 being the first standardized version. However, the 2000s led to a significant shift with the introduction of C++11.
Introduction to C++11 and C++14
C++11 marked a watershed moment in the evolution of C++. It introduced innovative features that fundamentally changed how developers approached coding. For instance, the addition of lambdas and smart pointers simplified complex tasks, making C++ much more versatile and programmer-friendly.
Following C++11, C++14 made further refinements and incorporated additional features, enhancing the language’s overall efficiency. Not only did these versions provide enhanced performance, but they also catered to the increasing demands of modern software development. The power of C++11 and C++14 features has since become vital for developers aiming to create robust applications efficiently.
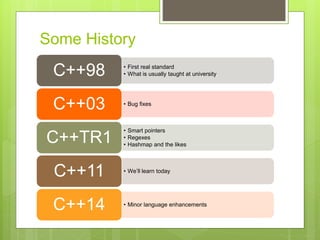
Key Features of C++11
Auto Keyword
One of the standout features introduced in C++11 is the auto keyword. This powerful addition allows the compiler to automatically deduce the type of a variable at compile time, significantly reducing the amount of code developers need to write. For example:
auto x = 5; // x is an int
auto y = 3.14; // y is a double
auto name = "C++"; // name is a const char*
This enhancement not only speeds up coding but also improves maintainability since you no longer need to specify the type explicitly.
Lambda Expressions
Another major addition is lambda expressions, which enable developers to write anonymous functions conveniently. Imagine wanting to sort a collection using custom criteria; with lambda expressions, you can write concise, readable code like this:
std::sort(vec.begin(), vec.end(), [](int a, int b) { return a < b; });
This feature transforms how algorithms work in C++, bringing functional programming principles right to the forefront.
Range-Based For Loop
C++11 also introduced the range-based for loop, making it easier to iterate over collections. No more traditional for loops with counters! You can now write cleaner and more intuitive code, such as:
for (const auto& element : collection) {
std::cout << element << " ";
}
This not only enhances readability but also reduces the chances of errors during iteration—another step toward leveraging the power of C++11 features.

Advanced Features of C++11
Smart Pointers
One of the significant advancements in C++11 is the introduction of smart pointers. These are automated memory management tools that help prevent common pitfalls like memory leaks and dangling pointers. With types like std::shared_ptr
, you can share ownership of an object:
std::shared_ptr<int> ptr1 = std::make_shared<int>(10);
std::shared_ptr<int> ptr2 = ptr1; // Both ptr1 and ptr2 own the memory
This feature means developers can focus on logic rather than manually managing memory.
Multithreading Support
C++11 greatly enhanced its capabilities with multithreading support. Developers can now execute multiple threads with ease, improving application performance. Using the <thread>
library, one can create and manage threads simply:
std::thread t([]{
std::cout << "Hello from thread!" << std::endl;
});
t.join(); // Wait for the thread to finish
This capability is crucial for modern software applications that require concurrency.
Move Semantics
Lastly, move semantics took C++ by storm, allowing resources to be transferred rather than copied. This means you can avoid unnecessary overhead and improve performance. Using std::move
, you can move resources efficiently:
std::vector<int> v1 = {1, 2, 3};
std::vector<int> v2 = std::move(v1); // v1 resources are moved to v2
This not only optimizes performance but also encourages better resource management. Collectively, these advanced features exemplify the power of C++11 in delivering efficient, high-performance applications.

Benefits and Usage of C++11 Features
Improved Performance
The advancements brought by C++11 significantly enhance application performance. With features like move semantics, resources are efficiently transferred without unnecessary copying, leading to faster execution times. This was evident in a project where I migrated legacy code to C++11; performance tests showed a notable speed-up when managing large data sets.
- Reduced Memory Usage: Smart pointers automatically manage memory, minimizing the risk of leaks.
- Concurrent Execution: Multithreading support allows developers to make the most of modern multi-core processors, executing tasks in parallel.
Enhanced Readability
C++11 not only boosts performance but also improves code readability. Features such as auto and lambda expressions encourage cleaner, more concise code. For example, using range-based for loops makes iterating through collections intuitive and straightforward:
for (const auto& item : collection) {
std::cout << item << " ";
}
This reduces clutter and helps both new and seasoned developers maintain and understand the code more easily.
Practical Examples
Implementing C++11 features in real-world applications unlocks numerous advantages. Take, for instance, a simple game engine; employing smart pointers can streamline memory management and significantly reduce crashes.
Additionally, multithreading can be harnessed to handle user inputs and game logic simultaneously, ensuring a seamless gaming experience. Overall, leveraging the power of C++11 features is a game-changer for developers aiming to create efficient, maintainable applications.

Introduction to C++14 Features
Generalized Lambda Capture
Building upon the foundation laid by C++11, C++14 introduced Generalized Lambda Capture. This feature allows developers to capture variables by move or copy directly within the lambda’s capture list. This flexibility can streamline code and improve performance, especially when handling mutable states. For example:
int x = 5;
auto lambda = [y = std::move(x)] { return y; };
This concise syntax enhances the expressiveness and efficiency of lambda expressions in your code.
Binary Literals
Another useful addition is binary literals, allowing programmers to define numeric values in binary format easily. This is particularly handy when dealing with flags or bitmasks. Instead of struggling with hexadecimal or decimal conversions, one can simply write:
int flags = 0b101010; // Represents the value 42 in binary
This makes it easier to visualize the bits of a number and improves code clarity.
Variable Templates
Lastly, C++14 also introduced Variable Templates, which provide a way to define templated variables. This feature simplifies various programming scenarios:
template<typename T>
constexpr T pi = T(3.1415926535897932385);
Such flexibility enhances consistency across your codebase, making repetitive tasks more manageable. Overall, these C++14 features not only improve coding efficiency but also bolster the usability of the language, paving the way for future innovations.
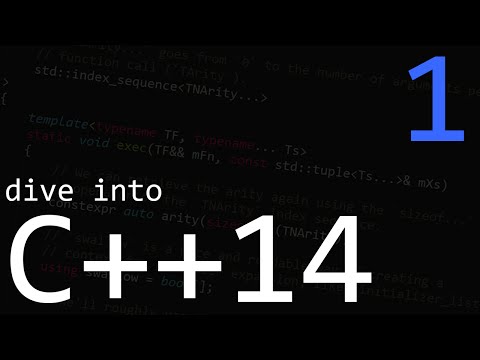
Enhanced Compile-Time Programming with C++14
constexpr Functions
C++14 took compile-time programming to the next level by enhancing constexpr functions. These functions can now contain a broader range of expressions, making it possible to compute values at compile time efficiently. For example, you can now use loops and conditionals within constexpr functions:
constexpr int factorial(int n) {
return (n <= 1) ? 1 : n * factorial(n - 1);
}
This capability can significantly improve performance, as many calculations that previously required runtime execution can now be done during compilation.
decltype(auto) Feature
Another exciting introduction is the decltype(auto) feature, which simplifies the process of declaring variables with inferred types. This can be particularly useful when returning types from functions that could vary depending on the input. For instance:
decltype(auto) getValue() {
int x = 10;
return x;
}
With decltype(auto), you capture the return type exactly as it appears, maintaining constness and references, which can eliminate potential pitfalls in type mismatches.
User-Defined Literals
Lastly, User-Defined Literals allow developers to define custom suffixes for their literals, which enhances code readability. For example:
constexpr long double operator"" _km(long double val) {
return val * 1000.0; // Convert kilometers to meters
}
This makes your code more intuitive, as you can use these literals to represent domain-specific concepts directly. Taken together, these enhancements in C++14 foster a more powerful programming environment, making compile-time computations and intuitive coding practices more accessible to developers.

Comparison of C++11 and C++14 Features
Similarities and Differences
When comparing C++11 and C++14, several similarities and differences emerge. Both versions focus on enhancing performance, readability, and developer productivity. However, C++14 builds upon the foundational principles laid by C++11, introducing refinements that enhance user experience.
Similarities include:
- Object-Oriented Programming: Both versions maintain strong support for OOP.
- Templates Usage: Advanced template programming remains a key feature.
Differences include:
- Enhanced Functionality: C++14’s
constexpr
functions can contain more complex statements, expanding compile-time computation capabilities. - New Syntax Features: C++14 introduced user-defined literals and generalized lambda captures, offering more flexibility than C++11.
Choosing the Right Version for Projects
Deciding which version to adopt for a project largely depends on specific requirements. If performance and modern features are paramount, C++14 is the better choice due to its enhanced capabilities. However, if working on an established codebase primarily written in C++11, the transition might require careful planning.
Ultimately, aligning with team familiarity and project goals is essential when choosing between C++11 and C++14. Embracing the power of both versions can significantly enhance overall development quality and efficiency.

Best Practices for Leveraging C++11 and C++14
Coding Guidelines
To maximize the benefits of C++11 and C++14, adhering to coding guidelines is crucial. Emphasizing readability and maintainability can significantly improve team collaboration. Consider:
- Using auto judiciously to simplify type declarations while keeping code clear.
- Implementing smart pointers where appropriate to manage resources efficiently, avoiding manual memory management.
- Prefer range-based for loops for cleaner iteration over collections.
Performance Optimization Tips
Optimizing performance is a key consideration when leveraging C++11 and C++14 features. Some practical tips include:
- Utilize move semantics to avoid unnecessary copying, particularly with large objects.
- Take advantage of multithreading capabilities to ensure your application fully utilizes available processor cores.
- Profile your application regularly to identify bottlenecks, allowing targeted optimizations where needed.
Learning Resources
Lastly, investing time in learning resources can greatly enhance your proficiency with C++11 and C++14. Websites like CPlusPlus.com and books such as “Effective Modern C++” by Scott Meyers provide insightful perspectives and practical guidelines. Engaging in coding communities or forums, like Stack Overflow and GitHub, can also provide real-world knowledge and support. Embracing these best practices will enable developers to harness the full power of C++11 and C++14 features while promoting an efficient, collaborative programming environment.

Future of C++ Programming
Upcoming Standards and Features
As we look towards the future of C++, several upcoming standards and features are generating excitement within the developer community. C++20 introduced significant enhancements, including concepts and ranges, simplifying template programming and making code more expressive. Looking further ahead, C++23 is expected to provide:
- More refined algorithms for collection manipulation.
- Pattern matching, enhancing the language’s expressiveness.
- Improved multidimensional array support, which is becoming increasingly vital in fields like data science.
These features are designed to address the evolving needs of developers, encouraging cleaner, more efficient code.
Adaptation and Industry Trends
The adaptation and industry trends surrounding C++ highlight its relevance in modern software development. Today, C++ is increasingly used in domains such as game development, high-performance computing, and systems programming. These industries demand low-level resource management and high execution speed—areas where C++ excels.
Moreover, companies are embracing modern C++ best practices, such as automated testing and continuous integration, to enhance software reliability and performance. As trends continue to evolve, C++ remains at the forefront, adapting to meet new challenges while retaining its core strengths. The future certainly looks bright for C++ programmers!