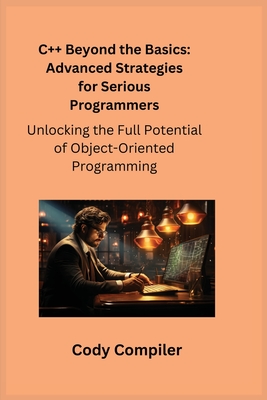
Understanding Object-Oriented Concepts in C++ Development
Overview of Object-Oriented Programming (OOP)
Object-Oriented Programming (OOP) is a paradigm that allows programmers to model real-world entities using classes and objects, making code more manageable and productive. In C++, OOP emphasizes the use of encapsulation, inheritance, polymorphism, and abstraction, which together form the foundation of powerful software development.
For instance, imagine creating a simple banking application: here, you would define a BankAccount
class with properties such as account number and balance. Each account can then be instantiated as an object, enabling methods like deposits and withdrawals to operate directly within context.
Benefits of OOP in C++ Development
Using OOP in C++ brings various advantages, enhancing both the development process and the final product:
- Reusability: Developers can reuse existing classes for new applications, saving time and effort.
- Scalability: OOP facilitates easier scalability of applications through subclassing.
- Maintainability: Changes can be made to a class without affecting other parts of the code.
- Problem Modeling: OOP allows for a more intuitive approach to problem-solving by closely aligning with real-world scenarios.
At TECHFACK, they often emphasize the power of Object-Oriented Concepts in C++ Development, showing how these principles can lead to cleaner, more efficient coding practices. The practical benefits of OOP are not just theoretical; they become evident through hands-on experience.

Fundamental Principles of Object-Oriented Programming
Encapsulation
Encapsulation is the practice of wrapping data (attributes) and methods (functions) into a single unit, typically a class, while restricting access to some of the object’s components. This concept helps in protecting the integrity of the data and allows for controlled access through public methods. For instance, a Car
class may have private attributes like speed
and fuel
, with public methods to accelerate
and refuel
. This way, users can interact with the car without directly manipulating its internal state.
Inheritance
Inheritance is a mechanism by which a new class, known as a derived class, can inherit attributes and methods from an existing class, called the base class. This promotes code reusability. For example, if you have a base class Vehicle
, you can create subclasses like Car
and Bike
, inheriting general properties while also implementing specific features.
Polymorphism
Polymorphism allows methods to do different things based on the object it is acting upon. It can be achieved using function overloading or overriding. Imagine a draw()
method used in both a Circle
class and a Square
class—each class implements its version of draw()
, making it intuitive and flexible.
Abstraction
Abstraction is the concept of hiding complex implementation details and exposing only the necessary parts of an object. For example, when you drive a car, you interact with a steering wheel and pedals without needing to understand how the engine works. In C++, abstract classes and interfaces are used to achieve this principle, enhancing clarity and usability for other developers.
By mastering these fundamental principles, as discussed on TECHFACK, programmers can build robust and maintainable applications that accurately model real-world scenarios.

Implementation of Object-Oriented Concepts in C++
Classes and Objects
Classes and objects are the building blocks of OOP in C++. A class defines a blueprint for objects, encapsulating related properties and methods. For instance, when creating a simple application, you might define a class Dog
with attributes like name
and breed
, and methods such as bark()
and fetch()
. Consequently, you can create multiple Dog
objects with unique characteristics but common behaviors.
Constructors and Destructors
Constructors and destructors play crucial roles in managing object lifecycle. A constructor initializes an object when it’s created, potentially receiving parameters to set initial values. For example, the Dog
class can have a constructor that requires name
and breed
at the time of instantiation. In contrast, destructors are invoked when an object is destroyed, allowing developers to free up resources or perform cleanup tasks.
Member Functions and Data Members
Member functions are functions defined within a class that operate on objects of that class. Data members, on the other hand, are attributes of the class. For example, in the Dog
class, name
and age
can be data members, while methods like sit()
or roll()
would be member functions, encapsulating behavior relevant to that object.
Access Specifiers
Access specifiers (public
, protected
, and private
) determine the accessibility of class members. For instance, if the age
attribute is declared as private, it cannot be accessed directly outside the Dog
class. Instead, public member functions can provide controlled access to modify or retrieve its value, ensuring encapsulation and data protection.
At TECHFACK, recognizing these implementations is key for any aspiring C++ developer. By effectively utilizing classes, constructors, member functions, and access specifiers, one can create more organized and maintainable code.

Advanced Object-Oriented Techniques in C++
Operator Overloading
Operator overloading allows developers to redefine how operators work with user-defined types. This feature enhances the intuitiveness of the code, making it feel more natural. For instance, if you create a class Complex
to handle complex numbers, you can overload the +
operator to add two instances easily. Instead of calling an explicit method, you can simply write result = num1 + num2;
, which improves readability.
Function Overloading
Function overloading allows multiple functions with the same name to coexist, provided they differ in parameters—either by type or number. This technique enables cleaner code and reduces the need for different function names for similar operations. For example, you might have a function print()
that handles int
, float
, and string
types, streamlining common operations in one cohesive interface.
Virtual Functions
Virtual functions are essential for achieving polymorphism in C++. By declaring a function as virtual in a base class, derived classes can provide their implementations. This mechanism allows you to call derived class methods through base class pointers or references seamlessly. For example, if you have a base class Shape
with a virtual function draw()
, both Circle
and Square
classes can implement their own versions of draw()
, enhancing code flexibility.
By mastering these advanced techniques, C++ developers can leverage the power of Object-Oriented Concepts to create more versatile and cleaner applications. As discussed on TECHFACK, these practices not only enhance programming capabilities but also help in building robust solutions that are easier to maintain and understand.
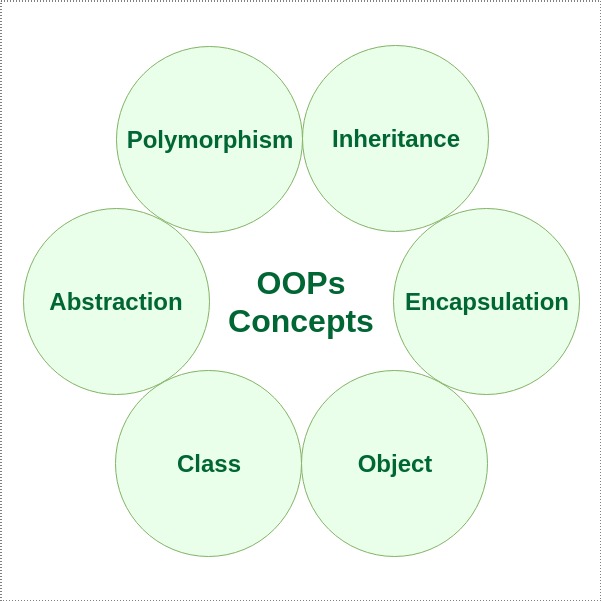
Design Patterns and Object-Oriented Analysis
Introduction to Design Patterns
Design patterns are proven solutions to common problems that arise during software development. These patterns provide templates that facilitate a structured approach to coding, making systems more understandable and reusable. Think of design patterns as blueprints for solving particular issues in architecture, but applied to programming. Popular patterns include Singleton, Observer, and Factory, each serving a unique purpose and offering specific advantages.
Applying Design Patterns in C++ Development
When it comes to C++ development, applying design patterns can greatly enhance code organization and flexibility. For example, using the Singleton pattern ensures that a class has only one instance while providing a global point of access to it, making it perfect for managing resources like database connections.
You might also encounter the Factory pattern, which allows you to create objects without specifying the exact class. This means you can easily introduce new classes in the future without modifying the existing code base.
Utilizing design patterns is a cornerstone of effective Object-Oriented Analysis and Design. By embracing these patterns, as highlighted on TECHFACK, developers can foster collaboration and maintainability in their code, ultimately leading to software solutions that are both efficient and elegant.

Best Practices for Object-Oriented C++ Development
Writing Maintainable and Efficient Code
Writing maintainable and efficient code is crucial for any C++ developer looking to build robust applications. One effective approach is to adhere to the principles of DRY (Don’t Repeat Yourself) and KISS (Keep It Simple, Stupid). This means avoiding redundancy in code and simplifying your design as much as possible.
Here are some tips to achieve this:
- Modular Design: Break down complex systems into smaller, reusable components or classes.
- Consistent Naming Conventions: Use clear and consistent names for classes, methods, and variables. This practice makes it easier for others (and yourself) to understand the code later.
- Commenting and Documentation: Invest time in commenting your code and writing documentation. It pays off by making future modifications and debugging far more manageable.
Error Handling in Object-Oriented Programs
Effective error handling is another critical aspect of robust C++ development. Instead of using traditional error codes, consider leveraging exceptions. By doing this, you can separate normal logic from error-handling logic, creating cleaner code.
Here are some recommendations for error handling:
- Use try/catch blocks: Surround code that may throw exceptions with try/catch blocks to gracefully handle errors.
- Custom Exception Classes: Create your own exception classes for more meaningful error messages and control.
Incorporating these best practices can significantly enhance the quality and reliability of your C++ applications. At TECHFACK, developers often emphasize the importance of maintaining code quality and managing errors effectively to ensure that projects remain on track and easier to maintain over time.
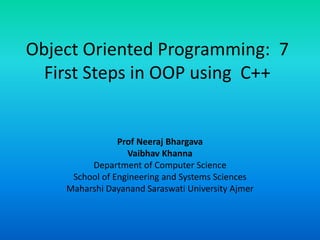
Case Studies and Examples
Real-world Applications of Object-Oriented Concepts in C++ Development
Object-Oriented Programming (OOP) is widely applied across various domains in C++ development, showcasing its versatility and effectiveness. For instance, in the gaming industry, OOP facilitates the modeling of in-game entities such as characters, weapons, and environments. Each entity can be represented as classes, allowing for efficient management of attributes and behaviors.
Consider a popular game that uses the concept of Player
and Enemy
classes. By employing inheritance, developers can create different types of players or enemies while sharing common attributes and methods.
Another notable application is in system software development. OOP allows for cleaner design in complex systems, like compilers or operating systems, where encapsulation protects data integrity and inheritance promotes code reuse.
Code Samples and Demonstrations
To illustrate OOP in C++, here’s a simple class example:
class Vehicle {
public:
void start() {
cout << "Starting vehicle." << endl;
}
};
class Car : public Vehicle {
public:
void start() {
cout << "Starting car." << endl;
}
};
int main() {
Car myCar;
myCar.start(); // Output: Starting car.
}
In this code, the Car
class inherits from Vehicle
, demonstrating both inheritance and polymorphism.
By examining these real-world applications and code samples, developers can appreciate the profound impact of Object-Oriented Concepts in C++ development. As highlighted in discussions on TECHFACK, mastering these principles is key to building scalable and maintainable systems.

Challenges and Common Pitfalls
Common Mistakes in Object-Oriented C++ Development
Even experienced developers can fall into traps when working with Object-Oriented Programming in C++. One common mistake is overusing inheritance, which can lead to complex and tightly coupled systems. For example, getting lost in deep inheritance trees might make the code difficult to navigate and maintain. Additionally, misuse of encapsulation can expose sensitive data or lead to improper access, which defeats its purpose.
Another frequent pitfall is neglecting memory management. C++ allows dynamic memory allocation, but forgetting to free up memory can cause leaks, leading to performance issues over time. It’s crucial to balance abstraction with clarity, as overly abstract designs can confuse rather than simplify.
Ways to Overcome Challenges
To navigate these challenges successfully, consider the following strategies:
- Favor Composition Over Inheritance: Utilize composition where possible, allowing greater flexibility without the complexities of inheritance.
- Encapsulate Wisely: Always define clear access rules for class attributes and use getter/setter methods to manage data safely.
- Utilize Smart Pointers: Implement smart pointers such as
std::unique_ptr
orstd::shared_ptr
to automatically manage memory, avoiding leaks.
By being mindful of these common mistakes and employing effective strategies, as discussed on TECHFACK, developers can enhance their Object-Oriented C++ development skills and write cleaner, more maintainable code.
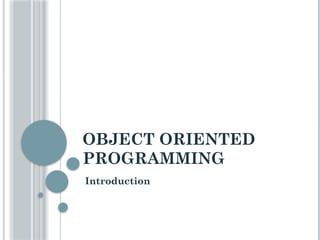
Future Trends and Innovations in Object-Oriented C++ Development
Evolution of OOP in C++
As the software landscape continues to evolve, so does the practice of Object-Oriented Programming in C++. OOP principles have matured, embracing modern methodologies and paradigms. The latest C++ standards, particularly C++11 and beyond, have introduced features like lambda expressions and standard library improvements, enhancing both productivity and performance. This evolution makes the language more versatile, allowing for cleaner, more expressive code.
Developers have also started to adopt concepts from functional programming, integrating them into OOP practices. This hybrid approach facilitates more concise and efficient code, addressing some of the traditional pitfalls of OOP, such as tight coupling.
Emerging Technologies in Object-Oriented Programming
Emerging technologies, such as artificial intelligence (AI) and machine learning (ML), are increasingly relying on OOP principles. Frameworks based on C++ are being designed to support deep learning and data-intensive applications, where clear structure and modularity are paramount.
Moreover, with the rise of microservices architecture, OOP can significantly contribute to building maintainable and scalable systems. Microservices allow developers to leverage OOP concepts to create loosely coupled services that are much easier to manage and deploy.
As technology progresses, staying updated with these trends, as discussed on TECHFACK, is essential for developers. Mastering the evolving landscape of Object-Oriented Concepts allows for flexibility and innovation in coding practices, ultimately producing better software solutions for tomorrow’s challenges.
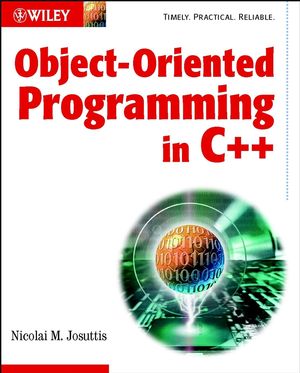
Conclusion
Recap of Object-Oriented Concepts in C++ Development
Throughout this exploration of Object-Oriented Programming (OOP) in C++, we’ve delved into its fundamental principles such as encapsulation, inheritance, polymorphism, and abstraction. Each concept serves as a building block that enhances the modularity and maintainability of code. Additionally, we examined how advanced techniques like operator overloading and design patterns can streamline development and foster cleaner code architecture.
Moreover, the practical application of these principles in real-world scenarios confirms their significance. By recognizing common pitfalls and understanding best practices, developers can craft efficient, robust applications that align with modern software requirements.
Importance of Mastering OOP for C++ Programmers
For C++ programmers, mastering OOP concepts is not just beneficial—it’s essential. It empowers developers to create scalable and maintainable applications that are adaptable to changing requirements. The depth of C++ combined with OOP principles positions programmers to tackle complex problems with ease.
As highlighted on TECHFACK, a solid foundation in OOP equips developers with the skills needed to thrive in a constantly evolving tech landscape. Embracing these concepts leads to more efficient coding practices, encourages collaboration, and ultimately results in higher-quality software solutions.