
Overview of Pointers in C++
Definition of Pointers
Now that we’ve set the stage for our discussion on C++ programming, let’s delve into the heart of the matter: pointers. A pointer in C++ is essentially a variable that stores the memory address of another variable. Think of it as a signpost pointing you in the direction of where a value lives in your computer’s memory. For instance, when you declare an integer variable, say int x;
, you can create a pointer to x
like this: int* p = &x;
, where &
denotes the address of x
.
Purpose and Benefits of Using Pointers
Pointers are powerful tools in C++ for a variety of reasons:
- Dynamic Memory Management: They allow developers to allocate memory at runtime, providing flexibility.
- Efficient Data Structures: Pointers enable the creation of complex data structures like linked lists and trees.
- Function Parameter Passing: Passing pointers to functions allows for efficient data manipulation without copying values.
I recall a project where using pointers significantly reduced memory overhead, improving performance in a complex algorithm. Embracing pointers can indeed enhance your programming experience!
Understanding pointers and memory management in C++ equips developers with the skills to write efficient, high-performance applications. Stay tuned as we explore memory management in the next section!
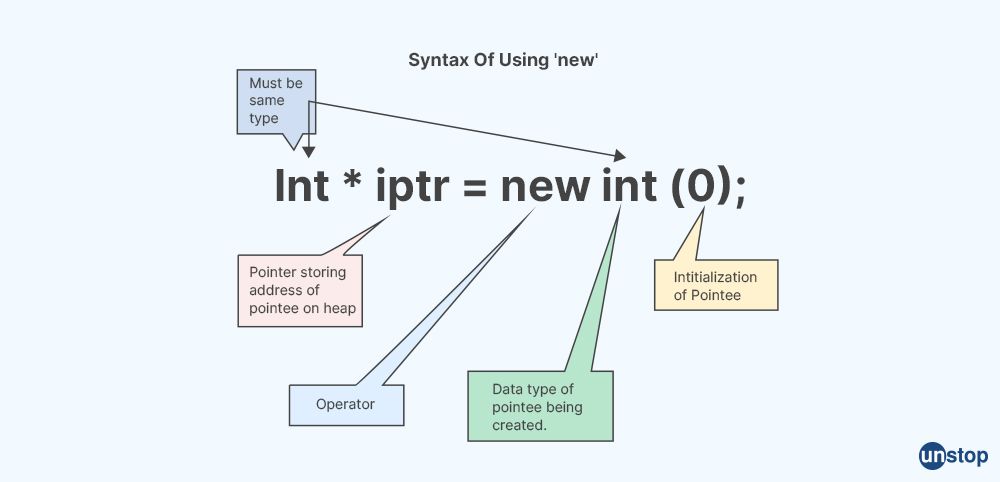
Memory Management in C++
Dynamic Memory Allocation
Building on our understanding of pointers, let’s dive into dynamic memory allocation, a crucial aspect of memory management in C++. Unlike static allocation, where memory size must be predetermined, dynamic allocation allows you to request memory during the runtime of your program. This is particularly useful for applications where data size cannot be estimated beforehand, like user-driven inputs.
You can allocate memory using the new
keyword. For example:
int* arr = new int[10]; // Allocates memory for an array of 10 integers
Allocation and Deallocation of Memory
Allocating memory is just part of the equation. Equally important is ensuring that memory is properly released. Neglecting to free allocated memory can lead to memory leaks, causing your application to consume more resources over time.
To deallocate memory, you use the delete
keyword:
delete[] arr; // Frees the allocated memory for the array
Here’s a quick checklist:
- Always match
new
withdelete
: If you allocate memory usingnew
, make sure you release it later withdelete
. - Use
delete[]
for arrays: This ensures each element is properly destructed.
In my experience, understanding dynamic memory allocation was a game-changer, especially when building performance-sensitive applications. It gives you unparalleled control over how memory is managed in your programs. Next up, we’ll explore foundational practices for working with pointers!
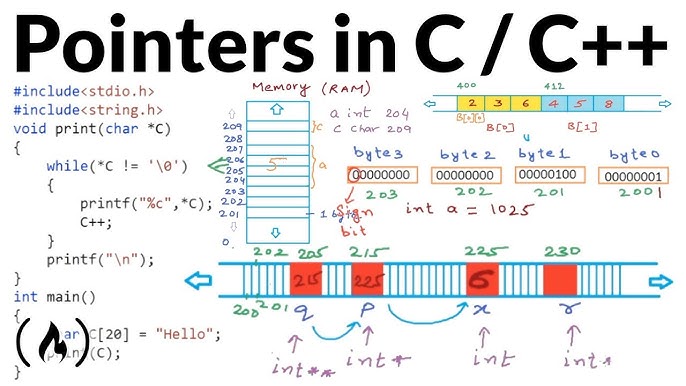
Working with Pointers
Declaring and Initializing Pointers
Having navigated through the intricacies of memory management, it’s time to get our hands dirty with pointers directly. Declaring a pointer is straightforward. You simply specify the type of data it will point to, followed by an asterisk (*) and the pointer’s name. Here’s an example:
int* ptr; // Declaration of a pointer to an integer
Initializing the pointer is equally important. You can assign it the address of a variable using the address-of operator (&):
int x = 5;
ptr = &x; // ptr now holds the address of x
This gives you the power to manipulate x
indirectly.
Dereferencing Pointers
Now that we have a pointer initialized, let’s talk about dereferencing. Dereferencing a pointer means accessing the value stored at the address the pointer is pointing to. You can achieve this using the dereference operator (*):
int value = *ptr; // value now holds the integer stored at the address ptr is pointing to
It’s almost like looking up the contents at the house that the pointer is pointing to!
In my coding journey, I often found dereferencing useful when passing large datasets to functions efficiently. Rather than copying data, I simply passed the pointer. This way, I could work directly with the data, improving efficiency without sacrificing clarity. As we press forward, we’ll explore important considerations regarding memory leaks and dangling pointers!
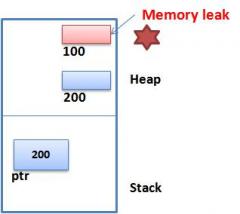
Memory Leaks and Dangling Pointers
Understanding Memory Leaks
As we continue our journey into pointers, it’s crucial to discuss memory leaks—perhaps the most notorious issue in memory management. A memory leak occurs when a program allocates memory dynamically but fails to deallocate it after use. Over time, this can lead to increased memory consumption and, ultimately, crashes, especially in long-running applications.
To visualize, imagine buying a new piece of furniture but neglecting to ever dispose of the old one; your space becomes cluttered over time.
- Common causes of memory leaks:
- Forgetting to use
delete
after usingnew
. - Losing reference to allocated memory (like reassigning a pointer).
- Forgetting to use
Here’s a proactive tip: Always track which memory blocks you’ve allocated, and match each new
with its corresponding delete
.
Identifying and Handling Dangling Pointers
Next, let’s talk about dangling pointers, which often arise from improper memory management. A dangling pointer refers to a pointer that still points to a memory location that has been deallocated. This can lead to unpredictable behavior or crashes, much like trying to use a key on a door that has been removed.
To handle dangling pointers effectively, take these precautions:
- Nullify pointers after deletion: For example, after using
delete
, set the pointer tonullptr
. - Avoid using pointers after the memory they refer to has been freed: Always check if a pointer is valid before dereferencing.
Reflecting on my experiences, implementing these practices has saved me from many headaches in debugging, ensuring cleaner and more efficient code. Up next, we’ll explore the intriguing world of pointer arithmetic and its practical applications!

Pointer Arithmetic
Incrementing and Decrementing Pointers
Having tackled the critical issues of memory leaks and dangling pointers, let’s dive into pointer arithmetic—a powerful feature of C++. Pointer arithmetic allows you to navigate through memory addresses with ease, akin to moving through a map.
When you increment a pointer (using ++
), it moves to the next memory address based on the type of data it points to. For example:
int arr[] = {10, 20, 30};
int* ptr = arr; // ptr points to the first element
ptr++; // Now ptr points to the second element: arr[1], which is 20
Similarly, decrementing a pointer (using --
) allows you to move backwards. This concept not only enhances readability but also simplifies code when working with arrays or list structures.
Pointer Arithmetic in Arrays
Arrays and pointers go hand in hand in C++. When you declare an array, the name of the array acts as a pointer to its first element. For instance, if you have an array called numbers
, accessing its elements can be done using either subscript notation or pointer arithmetic:
int numbers[] = {1, 2, 3, 4, 5};
int* ptr = numbers;
cout << *(ptr + 2); // This will output 3, the third element
From my experience, leveraging pointer arithmetic in arrays not only makes your code cleaner but also boosts performance, particularly in algorithms where you’re processing collections of data. As we continue, we’ll explore how pointers can work seamlessly with functions, enhancing our programming toolbox!
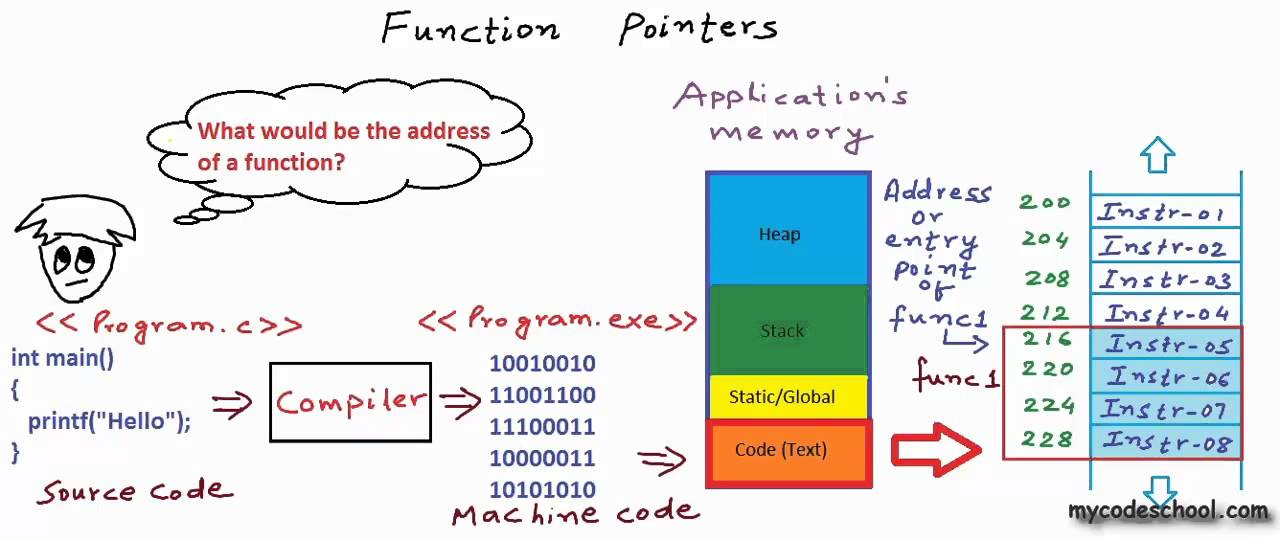
Pointers and Functions
Passing Pointers to Functions
As we delve further into pointers, let’s explore their integration with functions—an area where their power truly shines. Passing pointers to functions allows changes made within the function to affect the original variable. This approach is especially useful for manipulating large data sets without the overhead of copying them.
Consider this simple example:
void updateValue(int* ptr) {
*ptr = 20; // Change the value at the pointer’s address
}
int main() {
int x = 10;
updateValue(&x); // Passing the address of x
// Now x is 20
}
Using pointers in this way can lead to cleaner, more efficient… code.
Returning Pointers from Functions
Returning pointers from functions is another nifty trick in C++. When a function returns a pointer, it can provide access to dynamically allocated memory or specific data structures. However, this necessitates care to avoid dangling pointers.
Here’s an example where a function returns a pointer:
int* createArray(int size) {
return new int[size]; // Allocates an array dynamically
}
In my coding journey, returning pointers allowed me to build flexible data structures while managing memory efficiently. However, it’s crucial to remember to delete
the allocated memory later to avoid leaks.
With these tools, you’ll find yourself writing more robust and efficient programs. Next, we’re diving into advanced pointer concepts that will expand your understanding even further!
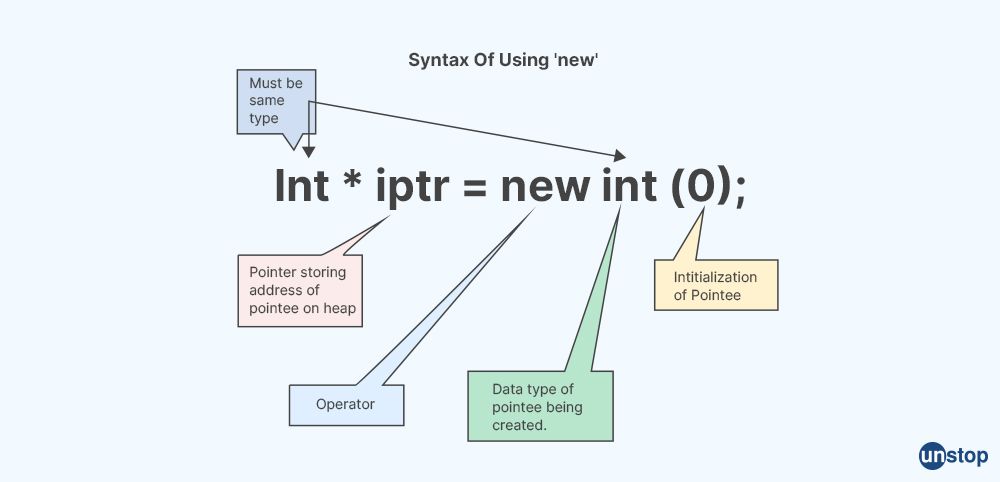
Advanced Pointer Concepts
Pointers to Pointers
Now that we’ve covered the essentials of pointers and functions, let’s explore some advanced concepts—starting with pointers to pointers. This might sound a bit complex at first, but think of it as a navigation system with multiple layers. A pointer to a pointer essentially holds the address of another pointer.
For instance, consider this example:
int x = 5;
int* ptr = &x; // Pointer to x
int** ptrToPtr = &ptr; // Pointer to ptr
When you dereference ptrToPtr
, you can access x
indirectly. This kind of structure is particularly useful in dynamic data management, such as when dealing with arrays of pointers or multi-dimensional arrays.
Pointers to Functions
Next up, let’s talk about pointers to functions. This concept allows you to pass functions as arguments or return them from other functions, enabling callbacks and more flexible program structures.
Here’s an example of defining and using a function pointer:
void display(int n) {
cout << "Value: " << n << endl;
}
void execute(void (*func)(int), int value) {
func(value); // Call the function via its pointer
}
Using function pointers can enhance your coding repertoire significantly. In one of my projects, I utilized function pointers to implement a simple event-driven system, and it made the design much more scalable and maintainable.
Embracing these advanced pointer concepts can lead to innovative solutions in your C++ programming journey. Up next, we will share best practices for memory management to ensure that your applications run smoothly and efficiently!

Best Practices for Memory Management
Avoiding Memory Leaks
Having explored advanced pointer concepts, it’s time to shift our focus to best practices for memory management—crucial for writing efficient and reliable C++ applications. One of the most pressing issues developers face is memory leaks, which can lead to wasted resources and program crashes if not handled properly.
To avoid memory leaks in your code, consider these best practices:
- Always match
new
withdelete
: Ensure that every memory allocation is appropriately freed. - Use smart pointers: C++ offers
std::unique_ptr
andstd::shared_ptr
, which automatically manage memory and mitigate leaks. - Regularly audit your memory usage: Utilize tools like Valgrind to identify leaks during development.
In a project I worked on, implementing smart pointers significantly reduced our debugging time and increased program stability.
Tips for Efficient Memory Allocation
Efficient memory allocation is another key consideration. Poor allocation strategies can lead to fragmentation and inefficient memory usage. Here are some tips to keep in mind:
- Allocate memory in chunks: For frequently requested sizes, consider using memory pools to reduce overhead.
- Avoid excessive allocations and deallocations: Reuse the same memory for different purposes when possible.
- Plan for growth: When allocating arrays, consider future needs and allocate a bit more space than currently required.
By implementing these strategies, you’ll enhance your coding efficiency, making your programs not only faster but also more reliable. Up next, we’ll discuss how to debug pointer-related issues effectively!
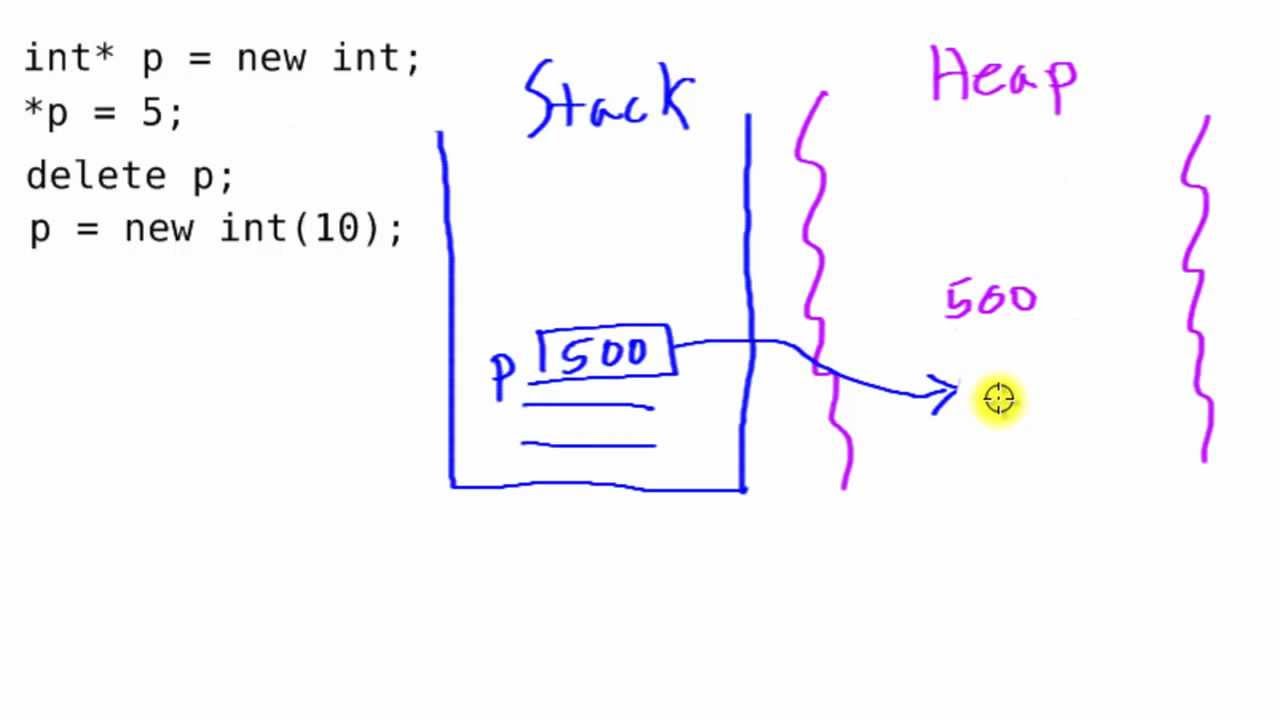
Debugging Pointer Related Issues
Common Pointer Errors
As we wrap up our exploration of pointers and memory management, it’s crucial to address the hiccups that can arise—primarily pointers related issues. Understanding common pointer errors can save you a lot of headaches later on. Here are a few typical culprits to watch out for:
- Dereferencing a null pointer: Attempting to access a value that the pointer points to zero leads to crashes.
- Dangling pointers: Trying to use a pointer that points to already-freed memory can lead to undefined behavior.
- Memory leaks: Not deallocating memory can accumulate over time, slowing down or crashing your application.
Throughout my coding journey, I’ve encountered these pitfalls more times than I’d like to admit, but identifying them early can make all the difference.
Tools for Debugging Pointer Issues
To tackle these issues effectively, there are several tools at your disposal:
- Valgrind: A powerful tool for detecting memory leaks and mismanagement.
- AddressSanitizer: An in-built compiler tool that catches misuse of memory, significantly reducing debugging time.
- GDB (GNU Debugger): Ideal for inspecting memory states and stepping through your code line by line.
Personally, incorporating Valgrind into my workflow transformed the way I tested my applications. By catching leaks and errors early, I spent less time in the debugging trenches and more time optimizing my code. With these tools and tips, you’re equipped to handle pointer-related challenges and build robust C++ applications!
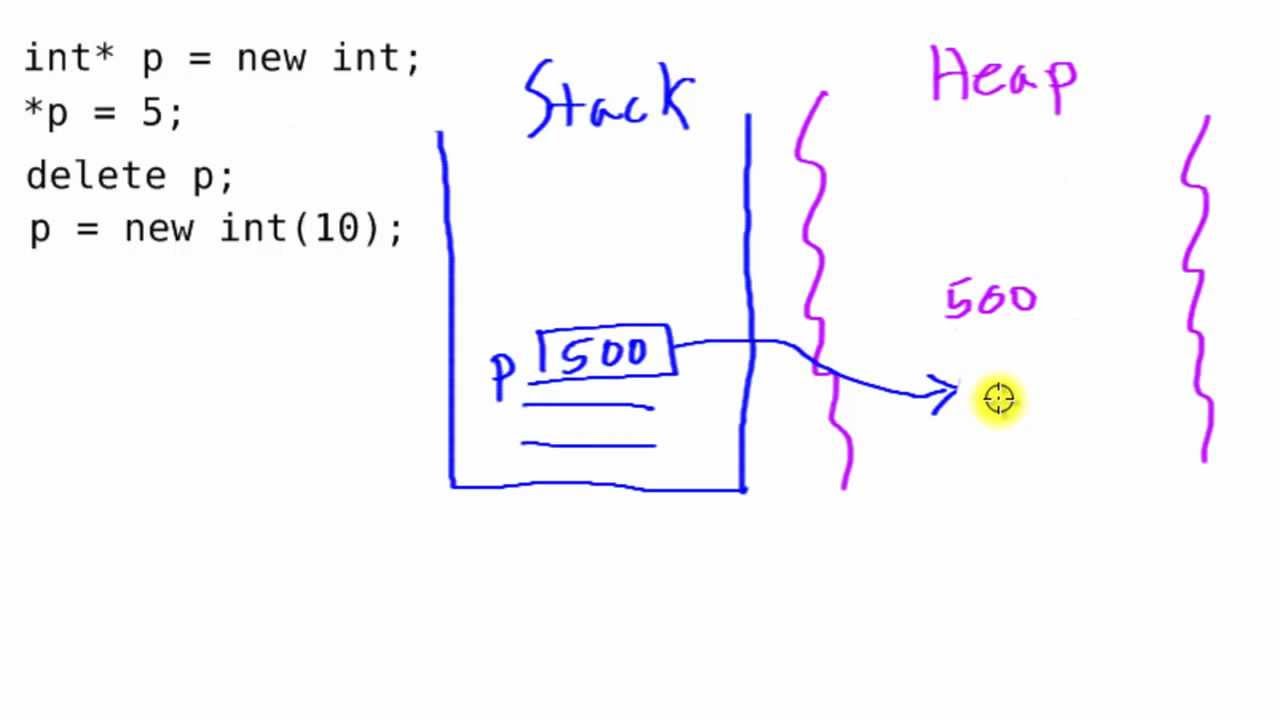
Conclusion
Recap of Key Concepts
As we conclude our deep dive into pointers and memory management in C++, it’s essential to recap the key concepts we’ve covered. We explored:
- Definition and Purpose of Pointers: Understanding how pointers work and their role in dynamic memory allocation.
- Memory Management Techniques: From avoiding leaks to efficiently allocating memory.
- Pointer Arithmetic: Learning how to increment, decrement, and navigate arrays using pointers.
- Function Pointers & Advanced Concepts: Gaining insights into pointers to pointers and pointers to functions.
- Debugging Pointers: Identifying common errors and utilizing tools like Valgrind for effective debugging.
Importance of Mastery in Pointers and Memory Management
Mastering pointers and memory management is crucial for any aspiring C++ developer. These skills not only enhance performance and resource management but also empower you to write more efficient and cleaner code.
Reflecting on my experiences, the deep understanding of these concepts has sometimes proven to be the difference between a functional program and a robust, high-performing solution. Embracing these principles allows developers to take full advantage of C++’s capabilities while mitigating potential pitfalls.
As you apply what you’ve learned, remember that continual practice and exploration are key. Your journey into the depths of C++ will undoubtedly lead to more innovative and successful projects!