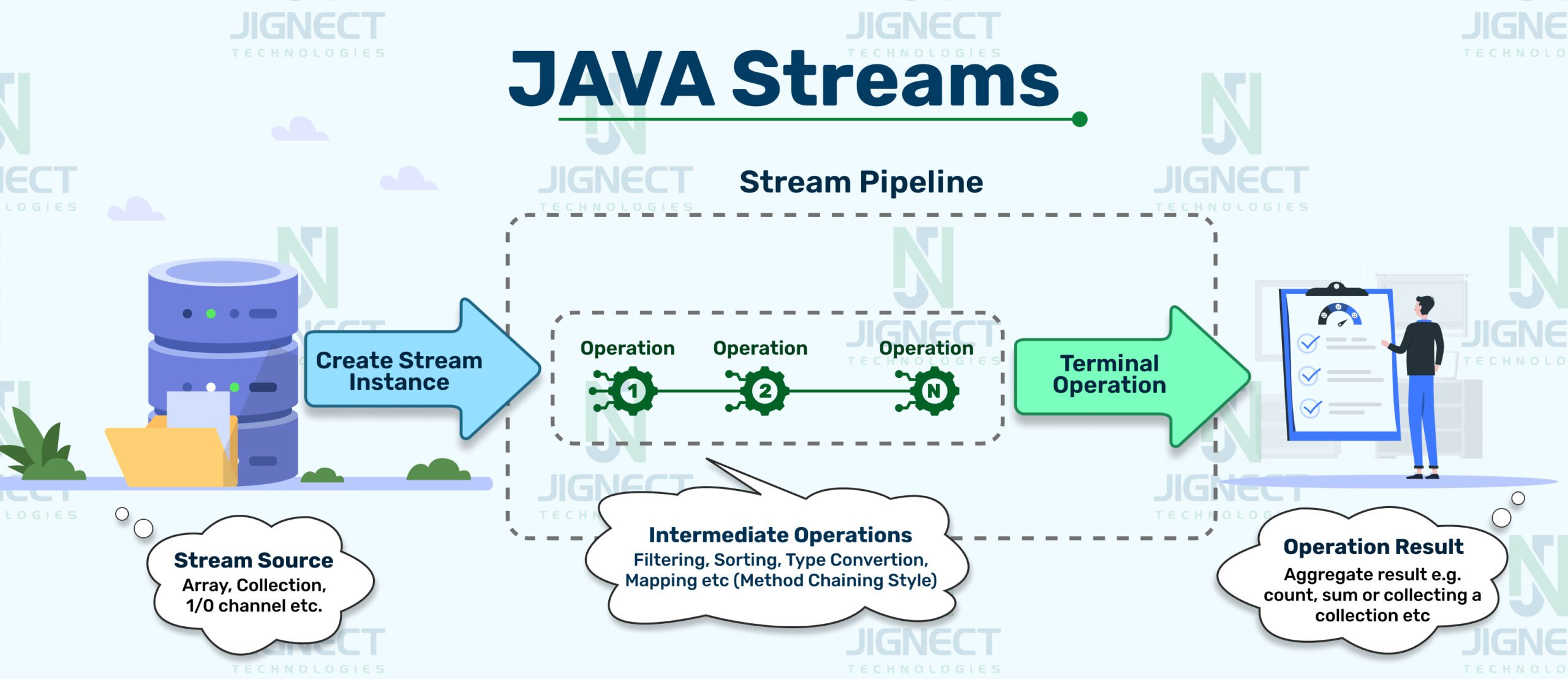
Streamlining Your Java Code: Data Manipulation in a Functional Style
When it comes to improving how we handle data in Java, embracing a functional programming style can significantly enhance code clarity and maintainability. By leveraging functional techniques, programmers can streamline their Java code, making it not only cleaner but also more efficient.
Emphasizing Immutability
One of the core principles of functional programming is immutability. It can be quite liberating to work with immutable data structures. Picture a scenario where you’re processing a list of customer transactions. Instead of modifying the original list, you create a new one holding the results. This approach not only keeps your data safe but also eliminates side effects:
- Preserved Data Integrity: Avoid unexpected changes to data.
- Easier Debugging: Bugs are less likely to arise from unintentional mutations.
Utilizing Functional Techniques
Functional programming provides powerful methods for data manipulation, such as:
- Higher-Order Functions: Functions that can accept or return other functions.
- Map, Filter, and Reduce: Techniques for transforming collections without explicit loops.
Imagine a situation where you’re filtering a list of users based on their activity level. With functional programming, this task can be achieved in a concise manner:
List<User> activeUsers = users.stream()
.filter(user -> user.isActive())
.collect(Collectors.toList());
At TECHFACK, we believe adopting a functional style in Java is not just a trend but a practical way to enhance productivity and streamline your Java code. So, why not take the plunge and rethink how you handle data manipulation with these approachable yet powerful strategies?

Streamlining Your Java Code: Data Manipulation in a Functional Style
Transitioning to functional programming in Java can be a game-changer for developers looking to enhance the way they manipulate data. It’s like discovering a more efficient toolbox for handling tasks that once seemed cumbersome.
Benefits of a Functional Approach
With functional programming, Java developers can write cleaner and more intuitive code. This style emphasizes functions as first-class citizens, allowing for more modular and reusable code snippets. Here are a few benefits that many developers, including myself, have experienced firsthand:
- Clarity and Readability: The code often reads more like plain English, which reduces the learning curve for newcomers.
- Easier Maintenance: Functional code tends to reduce state complexity and side effects, making it easier to spot and fix bugs.
Practical Example
To illustrate, consider processing a list of products to find which ones are in stock. Traditionally, this might involve lengthy loops and conditionals:
List<Product> inStock = new ArrayList<>();
for (Product product : products) {
if (product.isInStock()) {
inStock.add(product);
}
}
However, applying functional techniques simplifies this task:
List<Product> inStock = products.stream()
.filter(Product::isInStock)
.collect(Collectors.toList());
By adopting this functional style, tasks like data manipulation become smoother, enabling developers to focus on creativity rather than boilerplate code. At TECHFACK, we advocate for integrating these practices to elevate your coding experience, ensuring your Java code remains efficient and elegant.

Understanding Functional Programming
As programmers delve deeper into Java, one exciting approach that often surfaces is functional programming. This paradigm shifts the way developers think about code and data, and understanding its fundamentals can significantly enhance coding practices.
Introduction to Functional Programming
Functional programming is a programming style that treats computation as the evaluation of mathematical functions and avoids changing states and mutable data. Instead of focusing on how to perform tasks step-by-step (think imperative programming), functional programming emphasizes what to accomplish.
A personal experience comes to mind: when I first switched to functional programming, it felt like switching from a clunky old car to a sleek new model. Everything flowed smoothly, and I could focus more on solving problems rather than worrying about data management.
Benefits of Functional Programming in Java
Now, let’s dive into why adopting functional programming can be beneficial, especially in Java:
- Enhanced Readability: Code written in a functional style often reads more intuitively, making it easier for others (or your future self) to understand.
- Reduced Side Effects: By favoring immutability, functional programming minimizes unintended changes, leading to fewer bugs.
- Easier Testing and Maintenance: With pure functions (functions that always return the same output for the same input), testing becomes simpler. This drastically reduces the time spent debugging.
Adopting functional programming in Java can transform your coding approach, making it not only more enjoyable but also more effective. At TECHFACK, we’re excited to explore these concepts further and encourage you to give functional programming a try!
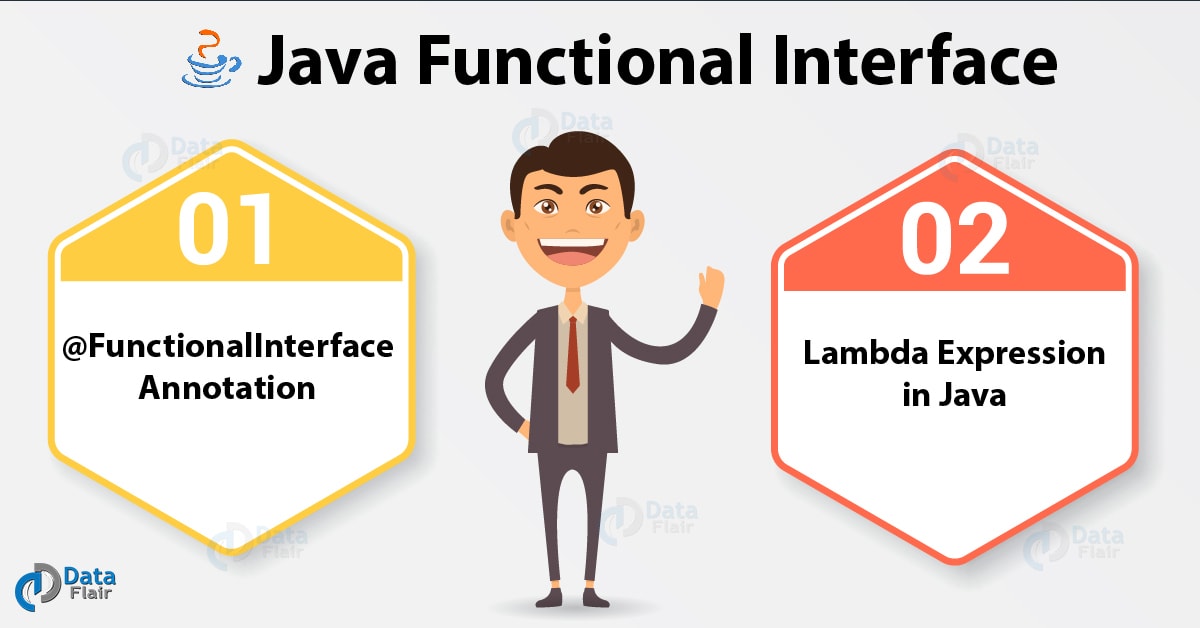
Basics of Data Manipulation
Having explored the foundations of functional programming, it’s essential to discuss how it applies to data manipulation in Java. Before diving into specific techniques, let’s clarify what data manipulation entails.
Overview of Data Manipulation in Java
Data manipulation refers to the process of managing and modifying data structures to prepare or analyze information effectively. In Java, this can involve working with collections such as lists, sets, maps, and arrays. With an ever-growing amount of data, knowing how to manipulate it efficiently is crucial.
I still remember the days when managing a large list of users involved cumbersome looping and manual updates. It felt cumbersome, almost like wading through thick mud. Those tasks can be streamlined significantly using modern techniques.
Functional Data Manipulation Techniques
So, how can we manipulate data functionally in Java? Here are some powerful techniques to consider:
- Streams API: This is perhaps the most significant feature introduced in Java 8. It allows developers to process sequences of elements in a functional manner. For example, transforming a list of numbers can be done in a single line.
- Map, Filter, and Reduce: These functional programming concepts are handy for transforming collections:
- Map: Applies a function to each element.
- Filter: Selects elements based on a predicate.
- Reduce: Combines elements into a single result.
Imagine filtering out inactive users from a list:
List<User> activeUsers = users.stream()
.filter(User::isActive)
.collect(Collectors.toList());
Incorporating these techniques can streamline your Java development experience, making data manipulation more intuitive and efficient. At TECHFACK, we encourage embracing these practices to enhance your coding toolkit!

Functional Interfaces and Lambda Expressions
Now that we’ve explored the essentials of data manipulation, let’s dive deeper into two powerful features of Java that make functional programming seamless: functional interfaces and lambda expressions. Understanding these concepts can dramatically enhance how you write and organize your code.
Exploring Functional Interfaces in Java
At the heart of functional programming in Java are functional interfaces. A functional interface is an interface that contains exactly one abstract method. This characteristic allows for clear and concise function definitions that can be implemented with lambda expressions.
For instance, consider a scenario where you need to perform operations such as sorting or filtering. Instead of defining a complete class for each operation, you can create a simple functional interface. Some popular functional interfaces provided by Java include:
- Predicate: Represents a function that takes an object of type T and returns a boolean.
- Function<T, R>: Takes an object of type T and returns an object of type R.
- Consumer: Accepts an object of type T and returns nothing.
Implementing Lambda Expressions for Data Manipulation
Once you have a grasp of functional interfaces, you can start utilizing lambda expressions to simplify your code. Lambda expressions allow you to create instances of functional interfaces in a more compact and expressive way.
For example, if you wanted to filter a list of integers, you could use a lambda expression as follows:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5, 6);
List<Integer> evenNumbers = numbers.stream()
.filter(n -> n % 2 == 0)
.collect(Collectors.toList());
This snippet effectively demonstrates how a lambda expression simplifies the filtering process by providing logic in-place without requiring multiple lines of boilerplate code.
At TECHFACK, we believe that mastering functional interfaces and lambda expressions can free developers from verbose coding practices while enhancing overall clarity. Embracing these tools opens up new possibilities for efficient and elegant code!
Stream API for Streamlining Data Processing
As we continue our exploration of functional programming in Java, one of the most transformative tools that developers have at their disposal is the Stream API. This powerful framework simplifies data processing and offers a more fluent way to work with collections.
Introduction to Stream API
The Stream API, introduced in Java 8, provides a high-level abstraction for processing sequences of elements (like collections) in a functional style. Think of it as a bridge that allows you to express complex data manipulations succinctly and efficiently.
When I first started using the Stream API, it felt like moving from a traditional manual transmission car to an automatic one—everything just flowed with ease! The core idea behind streams is to allow developers to write cleaner, more readable code by chaining operations on the data.
Streamlining Data Processing with Stream API
With the Stream API, you can perform various operations on collections, such as filtering, mapping, and reducing, all without writing cumbersome loops. Here are a few key functions you might find yourself using often:
- Filter: Removes unwanted elements.
- Map: Transforms each element in the stream.
- Collect: Gathers the result into a new collection.
For example, if you want to find the names of students who passed an exam from a list of students, you could write:
List<String> passingStudents = students.stream()
.filter(student -> student.getScore() >= 60)
.map(Student::getName)
.collect(Collectors.toList());
This simple line replaces prolific loops with clear, functional operations. At TECHFACK, we encourage embracing the Stream API to enhance your data processing practices, making complex tasks feel straightforward and manageable. The power of functional programming lies in these tools, and it’s time to harness them fully!