
Overview of Java Servlets and JSP
Introduction to Java Servlets
Java Servlets are a cornerstone of Java web applications, serving as the dynamic components that handle requests and responses between clients and servers. Think of them as the behind-the-scenes staff that ensure everything runs smoothly in a restaurant. When a customer (the client) places an order (the request), the server (the kitchen) relies on the servlet to cook and deliver the right dish (the response).
- Key Responsibilities of Java Servlets:
- Manage client requests and responses.
- Process form data and handle business logic.
- Interact with databases to retrieve or update information.
By using servlets, developers can create interactive applications that enhance user experiences. Remember the last time you filled out a contact form on a website? That’s a servlet in action, processing your information behind the curtain!
Understanding JavaServer Pages (JSP)
JavaServer Pages (JSP) complement servlets by allowing developers to create dynamic web content easily. While servlets generate HTML content programmatically, JSP simplifies this by embedding Java code directly into HTML pages, making it easier to manage and create user interfaces.
- Why Use JSP?
- Facilitates easier maintenance with a separation of presentation and logic.
- Offers tag libraries for rapid development and enhanced functionality.
- Streamlines the code required to render dynamic content.
Imagine building a web page where you want to display user-specific messages. With JSP, it becomes much simpler than writing extensive amounts of Java code in a servlet. It’s like using shortcuts when writing a story — easier and more efficient!
In conclusion, both Java Servlets and JSP are essential tools in the developer’s toolkit for building robust web applications, making web development not just effective but also enjoyable!

Advantages and Use Cases
Benefits of Java Servlets
Java Servlets come with a myriad of advantages that make them an attractive choice for web developers. One outstanding benefit is their ability to handle multiple requests simultaneously, which is essential for maintaining performance under heavy user loads. Think of it like a multitasking chef who can whip up multiple dishes at the same time without breaking a sweat!
Some key benefits of Java Servlets include:
- Platform Independence: Serve up your application on any server that supports the Java platform.
- Robustness: Built-in error handling and management capabilities increase reliability.
- Scalability: Efficiently manage the increasing number of requests as your user base grows.
These features position servlets as a fundamental building block in modern web development.
Common Use Cases for JSP
When it comes to JavaServer Pages (JSP), their primary role shines in creating dynamic content efficiently. They’re ideal for scenarios where visual appeal and user interaction are paramount, such as:
- E-commerce Applications: Personalizing the shopping experience based on user preferences.
- Blogs and Content Management Systems: Dynamically updating content without reloading the entire page.
- Dashboards: Presenting real-time data visualizations and analytics in an engaging manner.
Using JSP, developers can streamline the process of creating interactive web pages, ensuring that users enjoy a seamless experience. Just as a well-crafted menu makes a dining experience memorable, JSP enriches web applications with intuitive and dynamic interfaces.

Developing Servlets and JSP
Setting Up a Servlet Environment
Getting started with Java Servlets requires a well-configured environment. It’s like laying the groundwork before building a house. Here are the key steps you need to follow:
- Install Java Development Kit (JDK): Ensure you have the latest version to take advantage of new features.
- Choose a Servlet Container: Popular choices include Apache Tomcat and Jetty, which handle servlet requests and responses efficiently.
- Set Up a Development Environment: Use Integrated Development Environments (IDEs) such as Eclipse or IntelliJ IDEA for streamlined coding.
With everything set up, you’re ready to dive into servlet development!
Writing and Deploying Servlets
Writing a servlet is straightforward. You start by extending HttpServlet
and overriding key methods such as doGet
or doPost
to handle requests. Here’s a simple outline of what this looks like:
public class MyServlet extends HttpServlet {
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
response.getWriter().print("Hello, World!");
}
}
After writing your servlet, deploy it to your chosen servlet container. This process typically involves packaging your servlet into a .war
file and placing it in the webapps
directory.
Creating Dynamic Web Content with JSP
When it comes to JSP, creating dynamic web content becomes a breeze. Start by defining your HTML structure and embedding Java code using JSP tags. For example, you can easily incorporate Java logic to display a personalized welcome message:
<%@ page language="java" contentType="text/html; charset=UTF-8" pageEncoding="UTF-8"%>
<html>
<head>
<title>Welcome Page</title>
</head>
<body>
<h1>Welcome, <%= request.getParameter("username") %>!</h1>
</body>
</html>
Deploy your JSP files alongside your servlets, and watch as they work together to build engaging and responsive web applications. With these foundational skills in place, the world of Java web development is at your fingertips!

Handling Requests and Responses
Processing Client Requests
Once your servlet environment is set up and your servlets are written, the next critical step is handling client requests. Every time a user visits your website or submits a form, a request is sent to your server. Understanding how to process these requests is akin to understanding how to decode a customer’s order in a restaurant.
- Request Object: This is your primary tool for accessing client data. The
HttpServletRequest
object holds valuable information, such as:- User input (query parameters or form data)
- HTTP headers
- Session details
By calling methods like getParameter()
, developers can easily retrieve and leverage this data to customize the user experience.
Generating Server Responses
After processing the client’s request, it’s time to respond! The goal is to send information back to the user—whether that’s a confirmation message, data display, or an error notice.
- Response Object: Utilize the
HttpServletResponse
object to craft your reply. Here are some quick tips:- Set the content type using
setContentType()
, such astext/html
for web pages. - Write output using
PrintWriter
to send data back to the client.
- Set the content type using
For instance, after processing a form, you might want to show a success message:
response.setContentType("text/html");
PrintWriter out = response.getWriter();
out.println("<h1>Form Submitted Successfully!</h1>");
By adeptly managing requests and responses, developers can create seamless interactions that enhance user satisfaction, while keeping everything running smoothly behind the scenes.

Session Management and Cookies
Implementing Session Tracking
As we dive deeper into web applications, one of the most critical aspects is session management. Just like a customer at a restaurant expects the same waiter throughout their dining experience, users expect continuity while navigating a website. That’s where session tracking comes into play to maintain a user’s state across multiple requests.
- Creating a Session: In Java Servlets, you can create a session using the
HttpSession
object. This allows you to store user-specific data, making it easy to keep track of a user’s activity:HttpSession session = request.getSession(); session.setAttribute("username", "JohnDoe");
- Session Timeout: Manage session lifespan nicely. You can set a timeout to automatically invalidate the session after a certain period, enhancing security.
Working with Cookies in Servlets
Cookies are another essential tool for session management. They’re like small notes that your website gives your users to remember them. When a user visits your site, a cookie can store individual preferences or session identifiers.
- Creating and Retrieving Cookies:
- Use
javax.servlet.http.Cookie
to create a cookie:Cookie userCookie = new Cookie("username", "JohnDoe"); userCookie.setMaxAge(60 * 60 * 24); // 1 day response.addCookie(userCookie);
- Retrieve it later with:
Cookie[] cookies = request.getCookies(); for (Cookie cookie : cookies) { if (cookie.getName().equals("username")) { String username = cookie.getValue(); // Utilize the username as needed } }
- Use
By employing sessions and cookies effectively, developers can create a personalized user experience that keeps clients coming back, much like that loyal diner who always returns to their favorite eatery!

Java EE Technologies for Web Development
Overview of Java EE
Java EE (Enterprise Edition) is a robust platform specifically designed for developing large-scale, multi-tiered, distributed enterprise applications. It’s like having a versatile toolbox filled with everything a developer needs to build powerful applications that can withstand the pressures of real-world use.
- Key Features of Java EE:
- Standards-Based Framework: Enables developers to create applications that are portable across different environments.
- Scalability and Performance: Ideal for big organizations, supporting high volumes of transactions without skipping a beat.
- Integrated Services: Includes built-in support for features like messaging, persistence, and security.
Seeing the potential of Java EE, many businesses have leveraged its capabilities to streamline operations and drive growth.
Integrating Servlets and JSP in Enterprise Applications
When it comes to integrating Servlets and JSP within the Java EE framework, it allows developers to create dynamic, server-side applications that are both interactive and efficient.
- Seamless Interaction:
- Servlets handle the backend logic and data processing.
- JSP takes care of rendering the user interface, making it seamless to switch between handling complex data and presenting it beautifully.
For example, consider an online banking application where users view their transaction history. The servlet processes the user’s request, fetching data from the database, while the JSP formats that data into an easily digestible webpage.
Through this integration, developers can harness the combined strengths of Servlets and JSP to build scalable, maintainable, and dynamic enterprise applications that meet client needs. Embracing Java EE can truly elevate your web development efforts!

Best Practices and Security Measures
Secure Coding Practices
As developers build web applications using Java Servlets and JSP, adopting secure coding practices is crucial. Think of it as fortifying your digital castle to keep the bad actors at bay. Here are some essential practices to follow:
- Input Validation: Always validate user inputs to prevent harmful data from entering your application. Using whitelists (accepted input values) is preferable.
- Parameterized Queries: Utilize prepared statements to thwart SQL injection attacks. This practice ensures that any incoming data is treated as data, not code.
Example:
PreparedStatement stmt = connection.prepareStatement("SELECT * FROM users WHERE username=?");
stmt.setString(1, username);
- Error Handling: Avoid exposing sensitive data in error messages. Use generic error messages and log detailed errors privately.
Preventing Common Web Vulnerabilities
Even with secure coding practices, vulnerabilities can creep in. Being aware of common threats is half the battle. Here are a few to watch out for:
- Cross-Site Scripting (XSS): Always escape output data, especially user-generated content, to prevent malicious scripts from running in the user's browser.
- Cross-Site Request Forgery (CSRF): Implement CSRF tokens for forms to verify that requests originate from legitimate users.
By embedding these best practices into your development routine, you can significantly mitigate risks and enhance the overall security of your web applications. Remember, a proactive approach today helps prevent security headaches tomorrow!
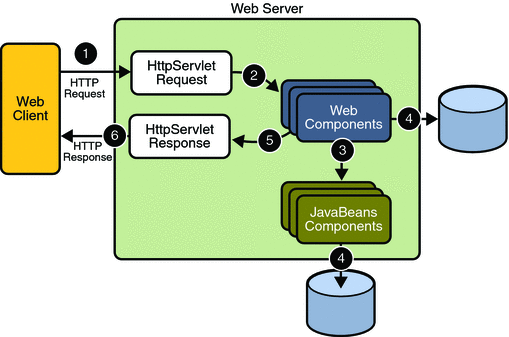
Deployment and Testing
Deploying Servlets and JSP Applications
Once you’ve developed and fine-tuned your Java Servlets and JSP applications, it’s time to deploy them. Think of this stage as hosting your grand opening after months of preparation! Here is a straightforward approach to ensure a smooth deployment:
- Package Your Application: Bundle your servlets and JSP files into a
.war
(Web Application Archive) file. This makes it easier to transfer your application to the server. - Choose Your Server: Common servlet containers include Apache Tomcat and GlassFish. Install your chosen server on any machine where you want to host your application.
- Deploy the
.war
File: Simply drop your packaged file into the server's designated deployment folder (likewebapps
in Tomcat), and restart the server. Voila! Your application is now live!
Testing Web Applications
With your application deployed, rigorous testing is key to ensuring everything functions smoothly. It’s like being a quality inspector before the grand opening.
- Functional Testing: Verify that every feature operates as intended. Create user scenarios to simulate real-world interactions.
- Performance Testing: Check how your application performs under stress. Tools like JMeter can help simulate multiple users and gauge response times.
- Security Testing: Scrutinize for vulnerabilities. Automated tools like OWASP ZAP can assist in identifying potential security flaws.
By meticulously deploying and testing your applications, you ensure not only a seamless user experience but also a robust and secure platform that stands resilient in the digital landscape!

Performance Optimization
Improving Servlet and JSP Performance
Once your Java Servlets and JSP applications are live, the next challenge is optimizing their performance. Think of it as tuning up a high-performance engine to ensure it runs smoothly and efficiently. Here are some strategies to consider:
- Minimize Initialization Time: Avoid heavy computations or database connections during servlet initialization. Instead, lazily load resources as needed.
- Connection Pooling: Use connection pooling to manage database connections effectively. It reduces overhead by reusing existing connections rather than creating new ones for each request.
Example (using Apache DBCP):
BasicDataSource dataSource = new BasicDataSource();
dataSource.setUrl("jdbc:mysql://localhost/db");
dataSource.setUsername("user");
dataSource.setPassword("password");
- Optimize JSP Compilation: Reduce the number of Java code snippets within JSP files. Extract complex logic to separate Java classes to improve readability and performance.
Caching Strategies for Web Applications
Implementing effective caching strategies can drastically improve your application’s response time. Caching temporarily stores frequently accessed data, reducing the need for repeated database queries.
- Use HTTP Caching: Leverage HTTP headers to allow browsers to cache static resources like images and stylesheets. This reduces load times on subsequent visits.
- Application-Level Caching: Tools like Ehcache can cache data objects in memory, enhancing performance significantly for data-intensive applications.
Implementing these performance optimization techniques will not only enhance user satisfaction with faster load times but also help your applications handle a larger user base efficiently. In the competitive landscape of web applications, performance can be a game-changer!