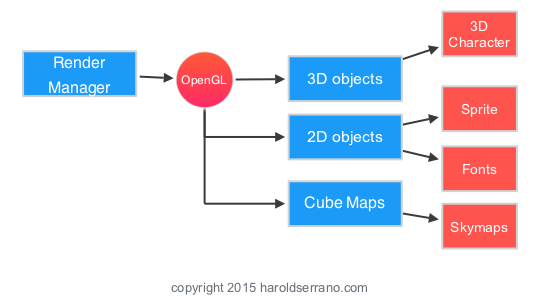
Overview of Game Engines
Definition of Game Engine
A game engine can be defined as a software framework that provides developers with the necessary tools to build and create video games efficiently. It acts as the backbone of a game, handling essential elements such as graphics rendering, physics simulation, audio processing, and user input management. By using a game engine, developers can focus on gameplay mechanics and storytelling without getting bogged down by low-level programming tasks.
Evolution of Game Engines
The journey of game engines is remarkable. Initially, game engines were simple and bespoke, crafted for single games. Over the years, they have evolved into powerful, reusable frameworks. Some key milestones include:
- Early Engines: Built specifically for particular games (e.g., Doom Engine).
- Portability: Rise of cross-platform engines (e.g., Unity, Unreal Engine).
- User-Friendly Tools: Emergence of visual scripting to democratize game development.
Importance of Building a Game Engine
Building a game engine has its unique advantages:
- Customization: Tailor the engine to meet specific project needs.
- Knowledge Acquisition: Gain a deeper understanding of game mechanics.
- Enhanced Performance: Optimize the engine for specific hardware.
By diving into game engine development, aspiring game creators can empower themselves to innovate in the gaming space. This insight can be invaluable for those looking to make a mark in the industry, something I can personally attest to from my own journey in game development.
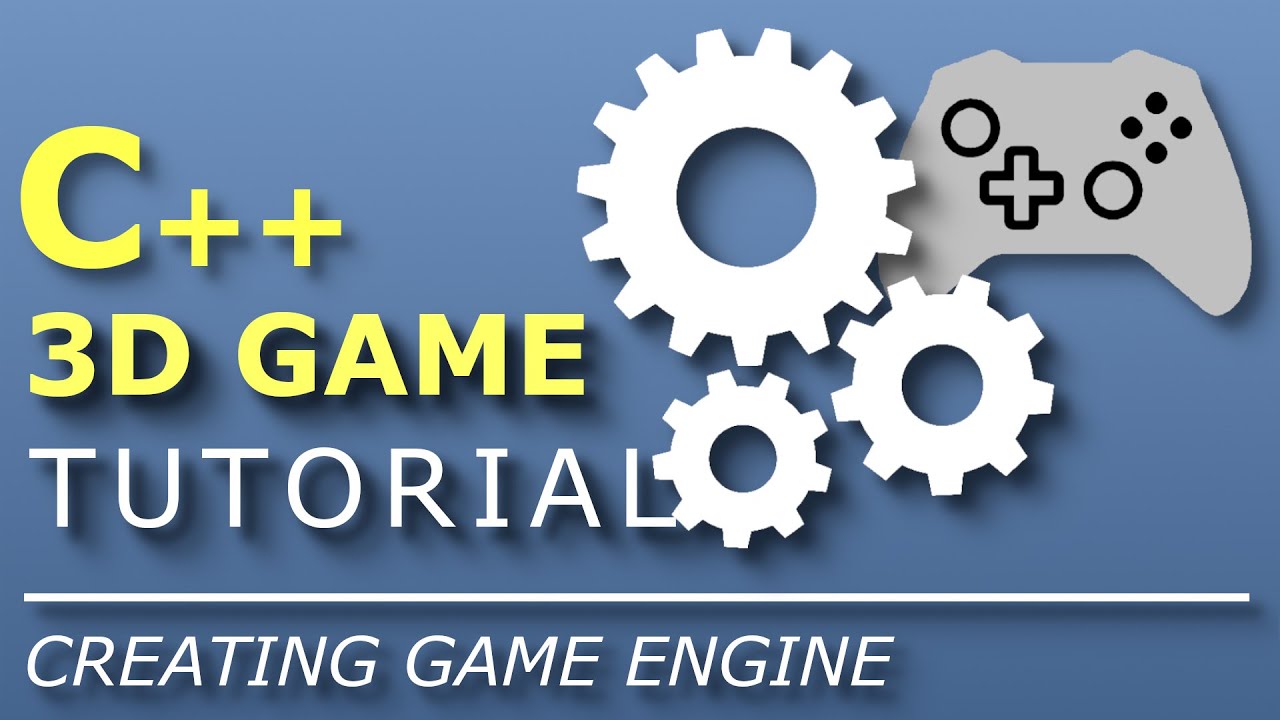
Introduction to C++ for Game Development
Basics of C++ Programming
When it comes to game development, C++ is a language that has stood the test of time and remains a favorite among developers. At its core, C++ offers powerful features like object-oriented programming, enabling developers to create modular and reusable code. By grasping the basics, you can quickly dive into more complex topics. Here’s what you need to know:
- Variables and Data Types: Understanding basic types like integers, floats, and strings.
- Control Structures: Mastery of loops and conditional statements.
- Functions and Scope: Utilizing functions to organize and reuse code.
C++ Features Relevant to Game Development
C++ provides distinct advantages that are vital for creating performance-driven games:
- Performance: Direct memory management allows for optimized execution speed.
- Low-Level Access: Control over hardware resources leads to better graphics and performance.
- Object-Oriented Design: Facilitates the creation of complex game systems through modular design.
By embracing C++, developers unlock powerful tools to create immersive gaming experiences, a realization I had early in my development career when I started seeing the capabilities in action.
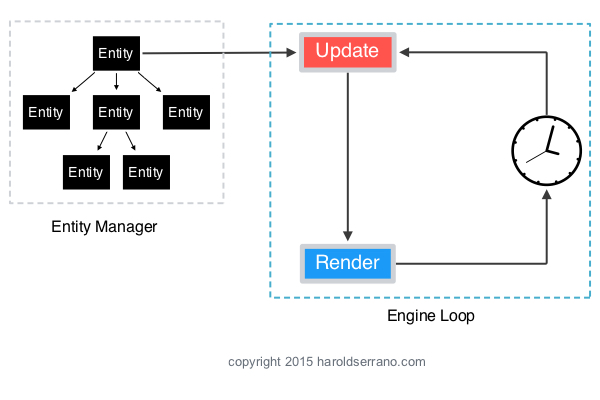
Planning Your Game Engine Architecture
Designing the Game Engine Structure
Once you grasp C++ basics, it’s time to focus on the architecture of your game engine. A well-designed structure is the foundation for a functional engine. Imagine it as the blueprint of a house; every component plays a critical role. Consider creating a modular design where different systems can operate independently. This allows for easier updates and maintenance. Key elements to consider include:
- Core Systems: Handle fundamental operations like rendering, input, and physics.
- Game Logic: Manage how game entities interact and behave over time.
Components of a Game Engine
Breaking down the components of a game engine further clarifies the design. Essential components include:
- Graphics Engine: Manages rendering and visual effects.
- Physics Engine: Simulates real-world physics.
- Audio System: Handles sound effects and music playback.
By planning carefully and understanding these components, developers can create a more efficient and dynamic game engine. This foresight has been instrumental in my own game projects, leading to smoother development cycles and enhanced gameplay experiences.
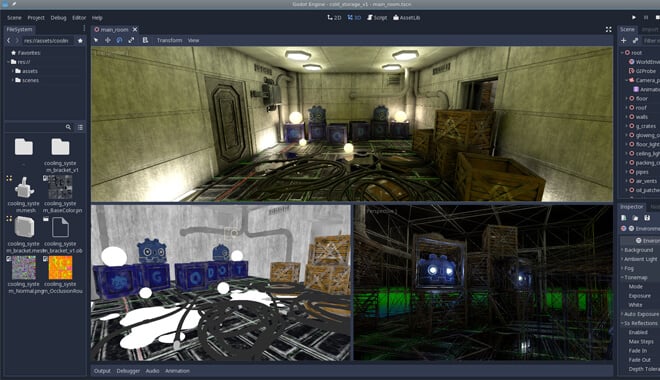
Setting Up the Development Environment
Installing Necessary Tools
With your game engine architecture in mind, it’s time to set up your development environment. This involves installing the necessary tools that will support your coding journey. Typical essentials include:
- C++ Compiler: Choose a reliable compiler like GCC or MSVC.
- Build System: Consider tools like CMake for managing project builds.
- Version Control: Tools such as Git to keep your code organized and manage changes.
From my experience, having these tools ready provides a solid foundation for writing and testing your code effectively.
Configuring Integrated Development Environment (IDE)
Next, let’s talk about configuring your Integrated Development Environment (IDE). A well-set IDE enhances productivity significantly. Recommended IDEs include:
- Visual Studio: Great for Windows development with powerful debugging tools.
- CLion: A cross-platform IDE with smart coding assistance.
Make sure to:
- Customize your workspace layout.
- Set up code highlighting and formatting preferences.
A properly-configured IDE can save hours of frustration, and this is something I cherish every time I dive into a new development project. Getting everything set up correctly is the first step toward realizing your game engine vision.

Implementing Core Systems in C++
Graphics Rendering System
Now that the development environment is set up, it’s time to implement some core systems essential for any game engine, starting with the graphics rendering system. This system is responsible for drawing everything that players see. Using libraries such as OpenGL or DirectX, developers can leverage C++ to create stunning visuals. Key steps include:
- Setting Up a Rendering Loop: This loop continuously updates the screen.
- Loading Textures and Models: Utilize efficient data structures for smooth rendering.
Physics Engine Integration
Next comes the physics engine integration. Realism is crucial in gaming, and a physics engine simulates how the game world behaves. You can choose to build one from scratch or integrate existing engines like Box2D or Bullet. Important considerations include:
- Collision Detection: Implement algorithms to determine interactions between objects.
- Rigid Body Dynamics: Simulate realistic movements and forces.
Input Management
Finally, input management is vital for player interaction. It captures keyboard, mouse, or gamepad events, translating them into game actions:
- Event Handling: Create event listeners to handle user inputs seamlessly.
- Mapping Controls: Design an intuitive control scheme that enhances user experience.
Establishing these core systems in C++ strengthens the foundation of your game engine, a realization I had when I first saw my rendered graphics come to life with responsive controls in my game projects. Each step adds layers of functionality that contribute significantly to overall player immersion.
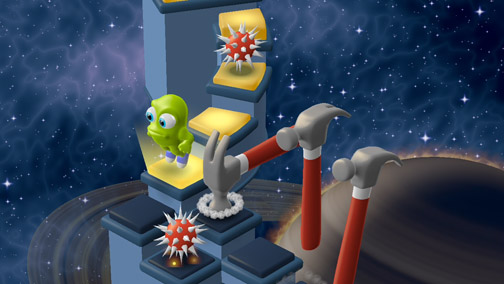
Incorporating Audio and Sound Systems
Handling Sound Effects
With core systems in place, it’s time to enhance the player’s experience by incorporating audio and sound systems. First up, handling sound effects. Sound effects bring a game to life, helping to create an immersive atmosphere. Utilizing libraries like FMOD or OpenAL, you can effectively manage sound files. Key steps include:
- Loading Sound Assets: Prepare various sound files, ensuring they are in compatible formats (e.g., WAV, MP3).
- Triggering Sounds: Implement event listeners to play sound effects based on in-game actions, like footsteps or explosions.
Integrating Music Playback
Next, integrating music playback adds another layer of depth to your game. Background music helps set the tone and evoke emotions. Here’s what to consider:
- Looping Tracks: Ensure background music can loop seamlessly to maintain immersion.
- Dynamic Music Changes: Implement transitions based on game events or player actions to enhance the experience further.
In my own development experience, the right audio elements transformed ordinary gameplay into captivating adventures, proving that audio is just as vital as visuals in creating memorable gaming moments.
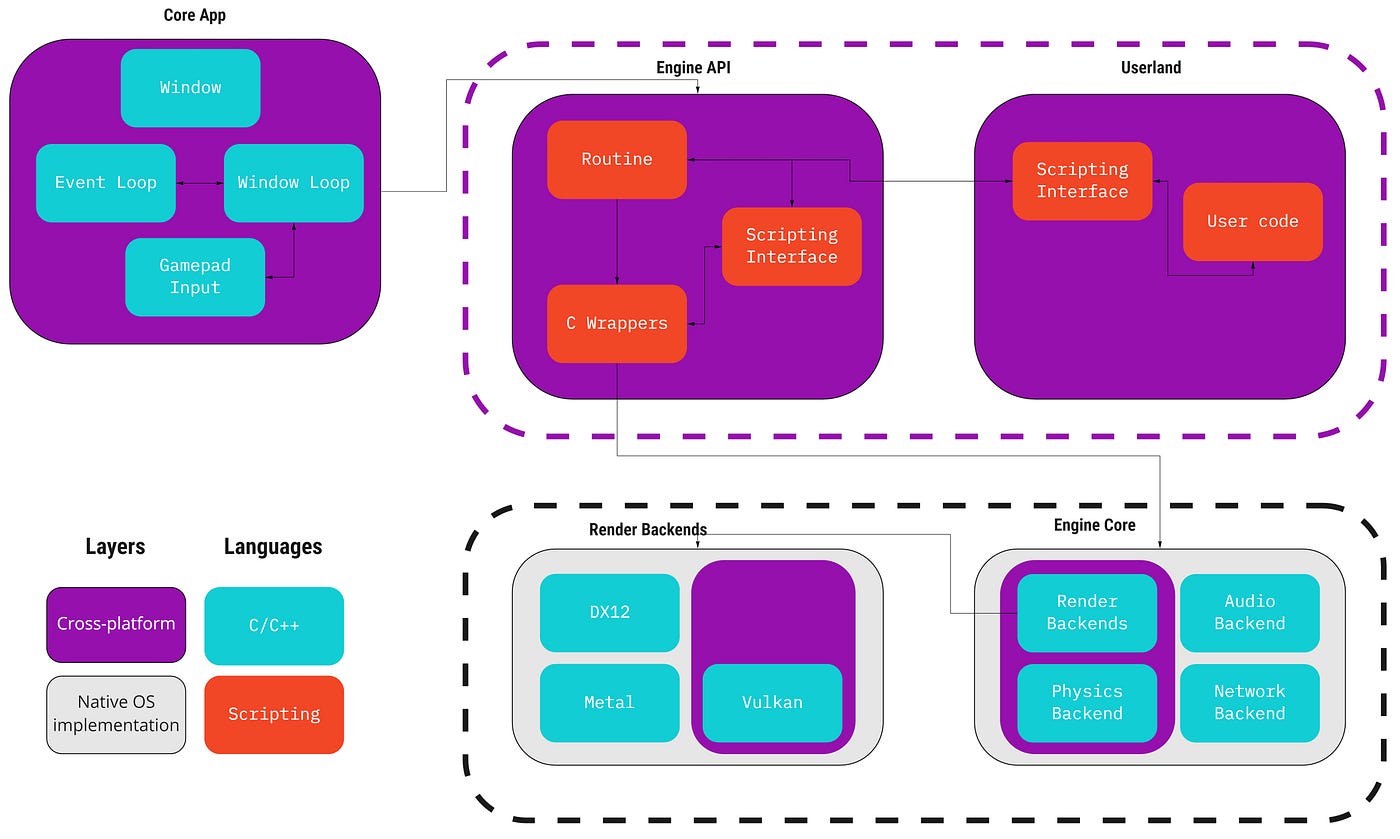
Adding Game Logic and Scripting Support
Implementing Game Behavior
With the audio and sound systems integrated, it’s time to add game logic and scripting support. Game behavior determines how characters and elements interact within the world. This is accomplished by defining rules and mechanics in your code. Here are some essential steps to consider:
- State Machines: Implement finite state machines (FSM) to manage different states of game entities, like idle, moving, or attacking.
- Event Systems: Create a robust event system to handle interactions, such as collisions or player input, which will drive game behavior.
Utilizing Scripting Languages
Incorporating scripting languages, such as Lua or Python, allows for flexibility and rapid iteration. Scripting can enable designers to tweak game mechanics without needing to recompile the C++ code. Benefits include:
- Easier Modifications: Non-programmers can change gameplay elements.
- Rapid Prototyping: Quickly test new ideas and tweaks.
In my game development journey, adopting scripting languages significantly improved collaboration with designers, allowing for a smoother workflow and fostering creativity in gameplay elements. This step is crucial for developing dynamic and engaging gaming experiences.
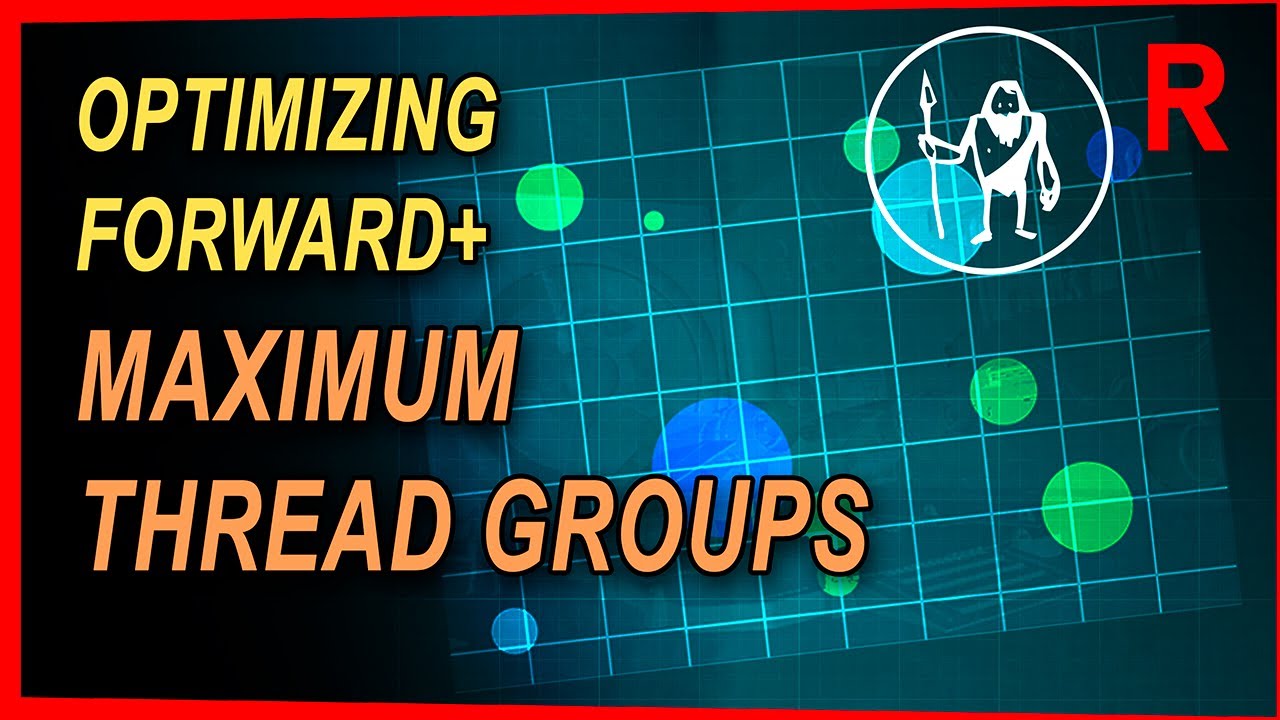
Optimizing Performance and Debugging
Profiling the Game Engine
After implementing game logic and scripting support, it’s crucial to focus on optimizing performance and debugging your game engine. Profiling the game engine helps identify bottlenecks and areas requiring improvement. Using tools like Visual Studio Profiler or gprof, you can monitor performance metrics such as:
- Frame Rate: Analyze how rendering affects gameplay.
- Memory Usage: Identify memory leaks or excessive allocations that may cause slowdowns.
From my experience, regular profiling allowed me to tweak performance settings early in development, saving me significant headaches later on.
Debugging Techniques for C++
Once optimization is underway, effective debugging techniques are essential to resolve issues quickly. C++ offers various strategies, including:
- Use of Debuggers: Tools like gdb or the integrated debugger in IDEs help trace and resolve errors.
- Logging: Implement logging frameworks to track game events and state changes.
In my own projects, I learned early that a thorough debugging process not only improves game stability but also provides insights into the underlying architecture. Optimizing performance and debugging becomes integral to delivering a polished and engaging gaming experience.
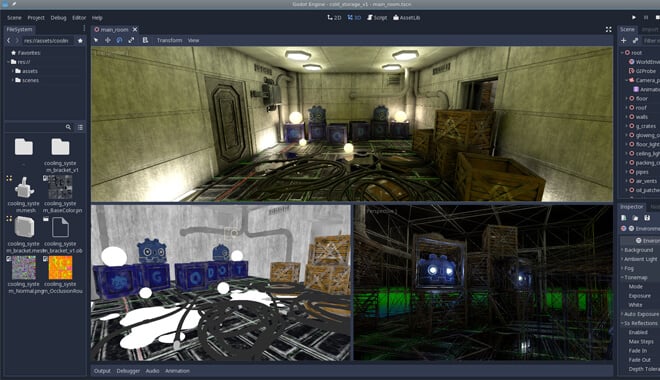
Testing and Refining Your Game Engine
Unit Testing Components
With optimization and debugging practices in place, it’s time to focus on testing and refining your game engine. Unit testing is crucial for ensuring that individual components work as intended. By writing tests for your game engine, you can catch issues early. Here are some tips for effective unit testing:
- Frameworks: Utilize testing frameworks like Google Test or Catch2 for organized test cases.
- Isolate Components: Test each function independently to ensure reliable behavior.
In my early projects, implementing unit tests not only improved stability but also boosted my confidence in making changes without fear of breaking existing features.
Iterative Development Process
Next, embrace an iterative development process. This approach allows you to refine your game engine progressively. Key aspects include:
- Regular Feedback: Gather input from playtesting to identify areas for improvement.
- Incremental Changes: Introduce features gradually, allowing for adjustments based on testing results.
Utilizing iterative development transformed my workflow, encouraging continuous improvements and leading to a more polished final product. This structured approach is vital in creating a robust and flexible game engine.
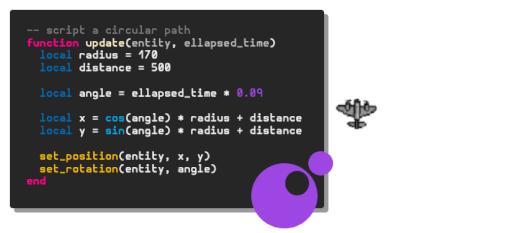
Deployment and Distribution
Compiling the Game Engine
With your game engine thoroughly tested and refined, the next step is deployment and distribution. The first crucial task is compiling the game engine. This process converts your C++ code into executable files that can run on target systems. Some steps to ensure a smooth compilation include:
- Using Build Tools: Leverage build systems like CMake or Makefile to streamline the compilation process.
- Optimization Flags: Apply optimization flags during the build to enhance performance.
In my experience, ensuring that my engine was compiled properly saved a lot of debugging time later on, as small inconsistencies can lead to major issues in performance.
Packaging for Different Platforms
Next, you’ll want to package your engine for different platforms. Catering to various operating systems (Windows, Mac, Linux) involves adjusting your packaging methods:
- Dependencies: Ensure that all required libraries and frameworks are included.
- Installer Creation: Use tools like Inno Setup or NSIS for creating user-friendly installers.
By packaging appropriately, you enhance user experience and broaden your audience. I’ve learned that taking these steps significantly impacts the reception of my projects, as a seamless installation process helps players dive straight into the game.
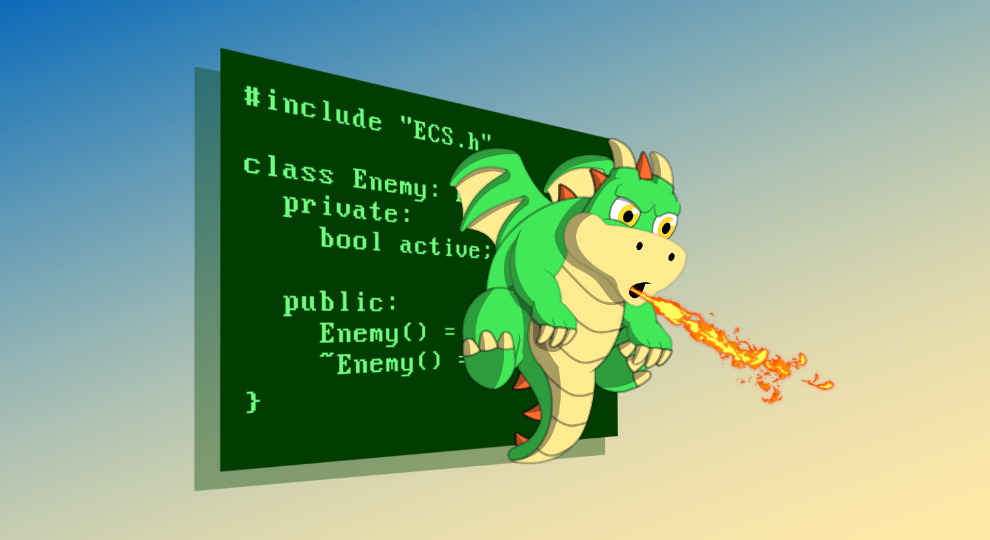
Community Support and Continued Development
Engaging with the Game Development Community
As you wrap up the deployment phase, it’s essential to leverage community support for ongoing development and innovation. Engaging with the game development community can provide invaluable insights, feedback, and networking opportunities. Here’s how you can get involved:
- Forums and Social Media: Join platforms like Reddit, Stack Overflow, or specialized game dev forums to share experiences and seek help.
- Game Jams: Participate in game jams to test your engine under pressure while connecting with other devs.
From my own journey, attending events and connecting with fellow developers greatly enriched my understanding and inspired new ideas for my projects.
Upgrading and Expanding the Game Engine功能
Lastly, as technology evolves, so should your game engine. Regularly upgrading and expanding its features keeps it relevant:
- New APIs and Libraries: Stay current with advancements in graphics and audio technologies.
- User Feedback: Use feedback from the community to prioritize which features to implement next.
By fostering a connection with the community and remaining adaptable, developers can ensure their game engine not only meets current standards but continues to thrive in an ever-changing landscape.