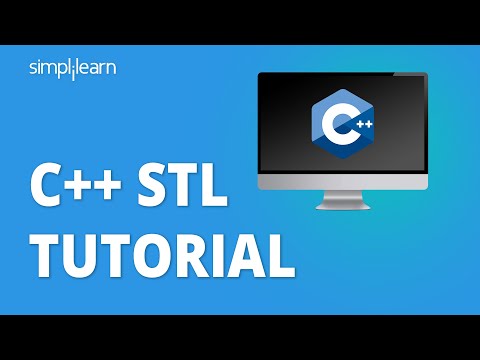
Introduction
What is C++ STL?
C++ Standard Template Library (STL) is a powerful collection of template classes and functions that provides ready-to-use data structures and algorithms. This library simplifies complex programming tasks by offering generic classes that enhance code flexibility and reusability. For instance, with STL, developers can handle collections of data like lists, arrays, and maps without needing to reinvent the wheel every time.
Why Learn C++ STL?
Learning C++ STL is essential for anyone looking to master C++. Here’s why:
- Efficiency: STL dramatically reduces development time by providing tried-and-tested algorithms and data structures.
- Performance: The operations designed in STL are optimized for speed and memory usage.
- Ease of Use: With straightforward syntax, you can accomplish complex tasks with minimal code.
As a budding programmer, discovering STL transformed how I approached problem-solving—allowing me to focus more on implementing logic rather than managing data structures.

Understanding the Basics of C++ STL
Containers in C++ STL
Containers are the backbone of the C++ STL, providing various ways to store collections of objects. Here are a few popular types of containers:
- Vectors: Dynamic array implementations that offer random access.
- Lists: Doubly linked lists that allow efficient insertions and deletions.
- Maps: Key-value pairs that facilitate data retrieval based on keys.
For example, I often choose vectors when I know the number of elements in advance, as they provide the performance I need.
Iterators in C++ STL
Iterators act as pointers that enable traversal through the elements of containers. They provide a uniform method to access container elements without exposing their underlying structure. Here are the main types of iterators:
- Input Iterators: Read elements from a container.
- Output Iterators: Write elements to a container.
- Bidirectional Iterators: Allow movement in both directions.
Understanding iterators is crucial, as they simplify data manipulation significantly.
Algorithms in C++ STL
C++ STL is rich in algorithms ranging from sorting and searching to modifying data. These algorithms work seamlessly with STL containers, making tasks simple and efficient. For instance:
- Sorting: Use
std::sort
to sort elements with ease. - Searching: Use
std::find
to locate elements within your containers.
When I first utilized std::sort
, I was amazed at how effortlessly I could sort my list of names with just one line of code, showcasing STL’s power in simplifying complex tasks.
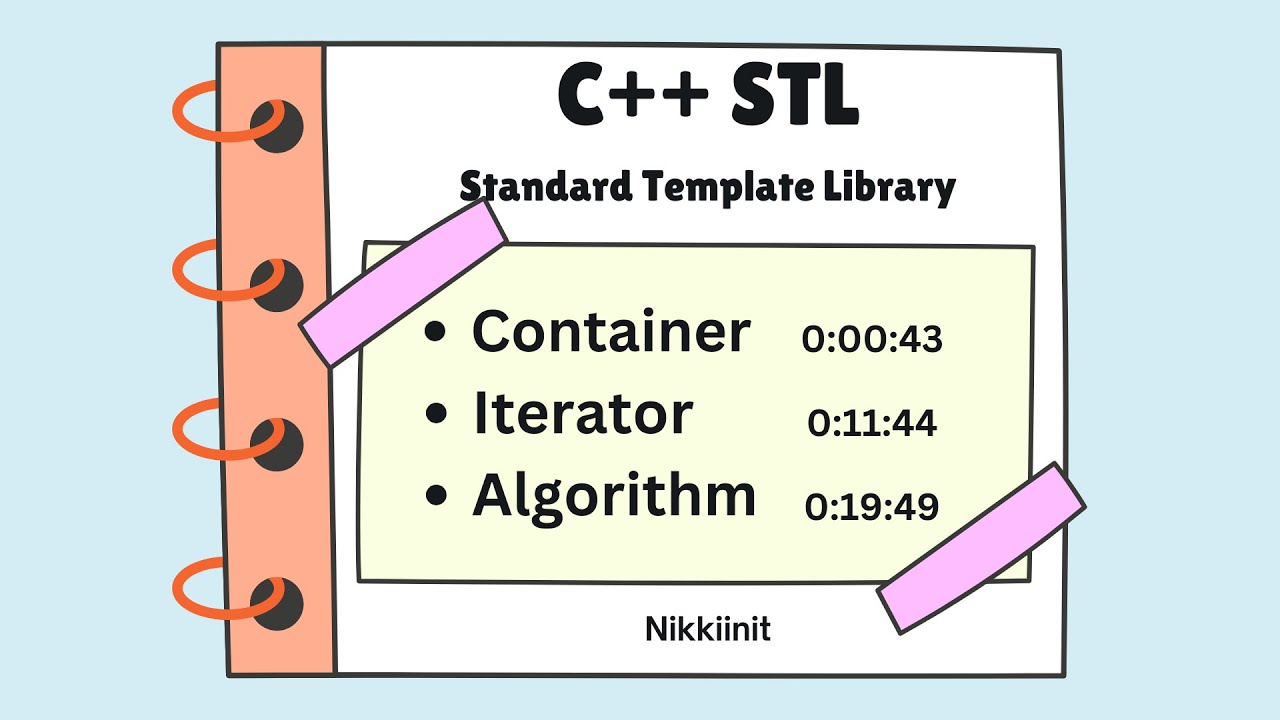
Working with Containers in C++ STL
Vector
One of the most versatile containers in C++ STL is the vector. Vectors are dynamic arrays that can resize automatically, accommodating elements as needed. They allow efficient access to elements by index, making them ideal for scenarios where you need quick lookups. For example, during a project to manage student records, I found vectors perfect for storing and accessing data quickly.
List
In contrast to vectors, lists are implemented as doubly linked lists, which excel in scenarios requiring frequent insertions and deletions. Lists allow for efficient splittings and concatenations, thanks to their structure. I often use lists when I need to manage a playlist of songs where users frequently add or remove tracks.
Map
Maps provide an associative array structure that stores key-value pairs, perfect for representing relationships between data. The unique keys allow for fast data retrieval and modification. When developing a contact management system, using maps to store names and phone numbers made lookups and updates straightforward.
Set
Sets are containers that store unique elements, ensuring no duplicates are present. They come in handy when maintaining a collection of unique items, like user IDs in a system. During my experience building a gaming leaderboard, sets allowed me to efficiently manage player usernames, preventing duplicate entries. Each of these containers plays a crucial role in data management, showcasing the flexibility of C++ STL.

Mastering Iterators in C++ STL
Input Iterators
Input iterators are the simplest form of iterators, designed exclusively for reading data from containers. They allow traversal in a single direction, which is ideal for accessing elements sequentially. While working on a reading application, I found input iterators extremely useful for processing user input line by line.
Output Iterators
Output iterators mirror input iterators but focus on writing data to containers instead. They enable inserting elements in a linear fashion. In one of my projects, I used output iterators to write formatted text to a vector, simplifying the script’s output management.
Forward Iterators
Forward iterators can read and write data, allowing movement only in one direction (forward). They are beneficial when you need to process each element without backtracking. I employed forward iterators for processing items in a shopping cart application, where I needed to calculate totals without revisiting items.
Bidirectional Iterators
Bidirectional iterators enhance forward iterators by allowing movement in both directions. This flexibility suits use cases like navigating a playlist where you might want to move back and forth between tracks. I remember using them to create a navigation feature for an online music app.
Random Access Iterators
Random access iterators provide the highest level of flexibility, enabling direct access to elements using indices, similar to arrays. They are ideal for operations requiring constant-time access. When developing a game, I utilized random access iterators for managing game objects, allowing swift updates and repositioning. Understanding these iterator types is essential for leveraging C++ STL effectively in various programming scenarios.
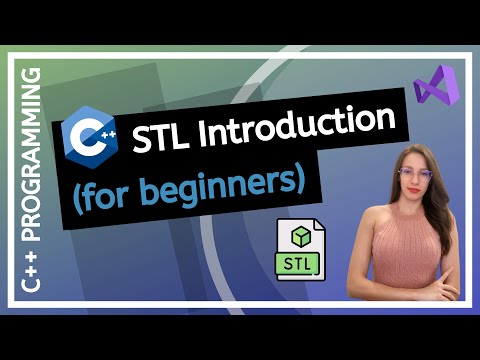
Implementing Algorithms in C++ STL
Sorting Algorithms
Sorting is one of the most common tasks in programming, and C++ STL provides powerful sorting algorithms that simplify the process. The std::sort
function, for instance, allows developers to sort data effortlessly with just one line of code. I recall needing to organize a list of user scores for a leaderboard; using std::sort
made it a breeze to rank the players quickly.
Searching Algorithms
Searching for elements in collections is just as straightforward with C++ STL. Functions like std::find
and std::binary_search
are optimized for efficiency. While working on a library management system, I utilized std::find
to quickly locate specific book titles, enhancing the user experience significantly.
Modifying Algorithms
Modifying algorithms, such as std::copy
, std::transform
, and std::remove
, enable developers to change collections efficiently. For instance, I often use std::transform
when I need to convert all text entries to uppercase in a user-input system—it allows for seamless transformations with minimal code.
Numeric Algorithms
Numeric algorithms in STL focus on mathematical operations like summation and accumulation. Functions such as std::accumulate
serve as handy tools for calculating totals or averages. I once utilized std::accumulate
to determine the total expenses for a project, proving how STL can handle complex tasks with elegance and simplicity. Embracing these algorithms not only speeds up coding but also enhances overall code readability and performance in C++ programming.

Advanced Topics in C++ STL
Function Objects
Function objects, or functors, are objects that can be treated as if they are functions. They overload the operator()
to allow easy invocation. I discovered the power of function objects while working on a filtering task where I needed to pass specific criteria to algorithms like std::remove_if
. This versatility not only keeps my code clean but also enhances performance.
Lambda Functions
Lambda functions are a fabulous feature introduced in C++11, allowing developers to define anonymous functions in a concise manner. They are particularly useful for short operations. I often use lambda functions for quick transformations, such as sorting or filtering collections. For example, filtering a list of integers to keep only even numbers became elegant and straightforward with a lambda expression.
Understanding Functors
Understanding functors is crucial as they provide significant flexibility in C++ programming. Unlike regular functions, functors can maintain state and offer more complex behavior. While developing a custom comparison function for sorting a structured dataset, I found defining a functor to encapsulate the comparison logic made the code easier to maintain and extend down the line. Utilizing these advanced topics significantly enhances both the functionality and clarity of C++ code, showcasing the depth of C++ STL’s capabilities.

Best Practices for Using C++ STL
Memory Management
Efficient memory management is pivotal when utilizing C++ STL. STL containers handle memory allocation and deallocation internally, but it’s essential to manage resources wisely. Always ensure that you’re using reserved space in containers like vectors to avoid excessive reallocations. Early in my career, I learned the hard way about performance hits from dynamic allocations by not reserving space for a frequently updated log of events, which slowed down my application considerably.
Exception Handling
C++ STL is robust, but exceptions can still arise, particularly with memory allocation or invalid accesses. Properly handling exceptions is crucial for maintaining application stability. I recommend using try-catch blocks around critical sections of code, especially when modifying or accessing STL containers. This practice allows you to address unexpected conditions gracefully and keep the user experience smooth.
Performance Considerations
When working with STL, always keep performance in mind. Use algorithms that suit your data structure, and prefer in-place algorithms when applicable to minimize overhead. For instance, I found that std::sort
was significantly faster for large datasets compared to a naive implementation. Additionally, profiling your code often leads to insights on where optimizations are most needed, ensuring your STL implementations remain efficient and effective in diverse scenarios. Adopting these best practices will enhance both the performance and reliability of your C++ projects, making you a more effective and proactive developer.

Conclusion
Recap of Key Learnings
Throughout this exploration of C++ STL, several key concepts emerged as foundational for efficient programming. We delved into:
- Containers: Understanding how to store and manage data effectively.
- Iterators: Learning the different types of iterators that enhance data traversal.
- Algorithms: Implementing sorting, searching, and numeric operations to manipulate data seamlessly.
- Advanced Topics: Exploring function objects, lambda functions, and functors for more elegant code.
Reflecting on these learnings, I realize how they have enriched my coding experiences and drastically improved my efficiency.
Next Steps in Mastering C++ STL
To continue mastering C++ STL, I recommend diving deeper into each topic through hands-on projects. Start with small tasks and progressively incorporate more complex structures and algorithms. Join coding communities or forums where you can share experiences and learn from others. Lastly, consistently practicing and contributing to open-source projects will not only solidify your understanding but also expose you to diverse applications of C++ STL in real-world scenarios. This ongoing journey will help you unlock the full power of C++ STL, as you continue to build robust and efficient applications in your career.