
Understanding the Basics of C++ Programming
Overview of C++ Language
C++ is a powerful, high-level programming language known for its efficiency and flexibility. Developed by Bjarne Stroustrup in the early 1980s, it combines the features of both low-level and high-level languages. C++ supports object-oriented programming (OOP) principles, making it an ideal choice for large applications requiring manageable and reusable code.
What sets C++ apart is its ability to handle complex tasks with ease, allowing developers to create a broad range of applications—from system software to games. Its compatibility with the C language facilitates the use of existing C codebases, offering a smooth transition for programmers.
Importance of Writing Efficient C++ Code
Writing efficient C++ code is crucial for several reasons:
- Performance: Efficient code runs faster, enhancing the user experience, especially in resource-intensive applications like gaming and simulations.
- Resource Management: Properly optimized code minimizes memory usage, which is essential in embedded systems and applications with limited resources.
- Maintenance: Cleaner, efficient code is easier to read and maintain, preventing future headaches during version upgrades.
For instance, while working on a graphics rendering project, I realized the difference that optimizing algorithms made; the application’s frame rate improved significantly, showcasing how critical efficiency is in real-world applications. By focusing on best practices for writing efficient C++ code, developers can greatly enhance the overall performance and longevity of their applications.
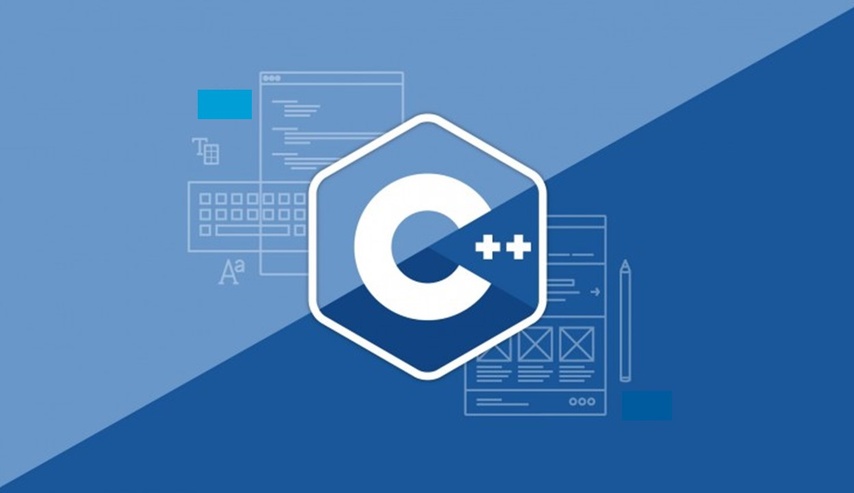
Performance Improvement Techniques
Efficient Memory Management
Efficient memory management is a cornerstone of writing high-performance C++ code. It involves optimizing how memory is allocated, used, and deallocated. Poor memory management can lead to bloat and leaks, slowing down applications or leading to crashes.
Here are some key strategies for efficient memory management:
- Use Smart Pointers: Instead of raw pointers, utilize smart pointers like
std::unique_ptr
andstd::shared_ptr
. They automatically handle memory cleanup, reducing the risk of leaks. - Reserve Capacity: When using containers like
std::vector
, reserve memory in advance if you know the required capacity, which can prevent unnecessary reallocations. - Avoid Global Variables: These can lead to increased memory usage and make it difficult to track memory allocations.
Avoiding Unnecessary Copying
Another critical technique for enhancing performance is avoiding unnecessary copying of objects. Copies are often expensive operations, especially for large objects. Emphasizing move semantics introduced in C++11 can substantially boost performance.
Consider these methods:
- Pass by Reference: Instead of passing objects to functions by value, pass them by reference or const reference.
- Move Semantics: Leverage move constructors and move assignment operators to transfer resources rather than copying them.
For example, in a recent project involving a data processing application, I switched from passing large data structures by value to using references and noted a dramatic improvement in performance. By focusing on efficient memory management and avoiding unnecessary copying, C++ developers can ensure their applications run smoothly and efficiently.

Proper Utilization of Standard Library
Using Standard Algorithms
One of the most powerful features of C++ is its Standard Library, which offers a rich set of algorithms designed to streamline your coding process. Using these built-in algorithms can enhance efficiency significantly. For instance, instead of writing your own sorting or searching functions, you can leverage algorithms like std::sort
and std::find
.
Benefits of using standard algorithms include:
- Optimized Performance: These algorithms are tailored to be efficient for a wide range of scenarios.
- Code Clarity: Using descriptive algorithm names makes your intentions clear to anyone reading your code. It enhances maintainability.
In my experience, adopting standard algorithms after a cluttered, custom solution for a sorting problem made a world of difference. Not only was my code shorter, but it also ran faster.
Leveraging Containers Effectively
C++ offers various containers in its Standard Library, like std::vector
, std::list
, and std::map
, each suited for different use cases. Choosing the right container can lead to significant performance improvements.
Consider these tips:
- Select Based on Use Case: For random access, prefer
std::vector
; for frequent insertions/deletions,std::list
is more suitable. - Utilize Appropriate Operations: Familiarize yourself with container-specific operations to maximize performance.
By utilizing the standard library effectively, developers can save time and resources, leading to a more efficient coding experience. Integrating standard algorithms and containers can truly elevate the quality of C++ applications.
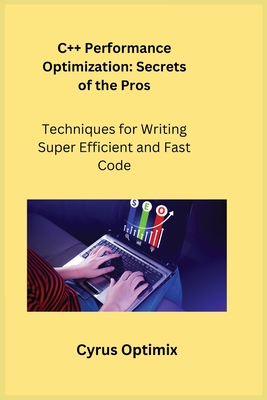
Optimizing Code for Speed and Efficiency
Loop Optimization Techniques
Loops are fundamental in programming, but they can also become bottlenecks if not optimized properly. Adopting effective loop optimization techniques can dramatically enhance the performance of your C++ code. Here are some key strategies:
- Unrolling Loops: By manually unrolling loops, you can reduce the overhead of loop control, which may lead to improved performance. For instance, instead of incrementing a variable for each iteration, process multiple elements per iteration.
- Minimize Loop Overhead: Whenever possible, move calculations or function calls outside the loop. This can streamline the loop and eliminate unnecessary computations.
In one of my projects involving heavy data processing, implementing these optimizations turned a sluggish loop into a much faster operation, significantly improving the overall execution time.
Minimizing Function Calls
Function calls introduce overhead due to stack operations and context switching. To optimize speed, it’s essential to minimize unnecessary function calls.
Consider these approaches:
- Inline Functions: For small functions, using the
inline
keyword allows the compiler to replace the function call with the actual function code, reducing call overhead. - Combine Functions: When related operations are done sequentially, combining them into a single function can reduce the number of calls and enhance performance.
By focusing on optimizing loops and minimizing function calls, developers can achieve significant performance gains, making C++ applications not only faster but also more efficient. The importance of these techniques can’t be overstated, especially when working on large-scale projects where every millisecond counts.

Utilizing Compiler Optimizations
Understanding Compiler Flags
Compiler flags are powerful tools that instruct the compiler on how to optimize the code during the build process. By carefully selecting the right flags, developers can enhance the performance of their C++ applications significantly.
Some essential compiler flags to consider include:
- -O1, -O2, -O3: These flags enable different levels of optimization, with -O3 providing the highest level. While higher optimization levels can yield faster code, they may also increase compile time.
- -march=native: This flag allows the compiler to optimize the code for the specific architecture of the machine, taking full advantage of its instruction set.
In a project I was involved with, switching to the -O2
optimization flag resulted in noticeable improvements in runtime efficiency, demonstrating the power of compiler flags.
Compiler-Specific Optimization Techniques
Different compilers, like GCC, Clang, and MSVC, have their own sets of optimization techniques. Understanding these specific options can unlock additional performance capabilities:
- Profile-Guided Optimization (PGO): This technique collects data about the program’s usage at runtime and uses this information to optimize the final build. It can lead to significant speedups, particularly in large applications.
- Link-time Optimization (LTO): This compiles and optimizes across translation units, allowing for more global optimizations.
By leveraging compiler optimizations, developers can significantly enhance the performance of C++ applications, ensuring they run efficiently in diverse environments. This level of attention to optimization can make a profound difference to the final user experience.
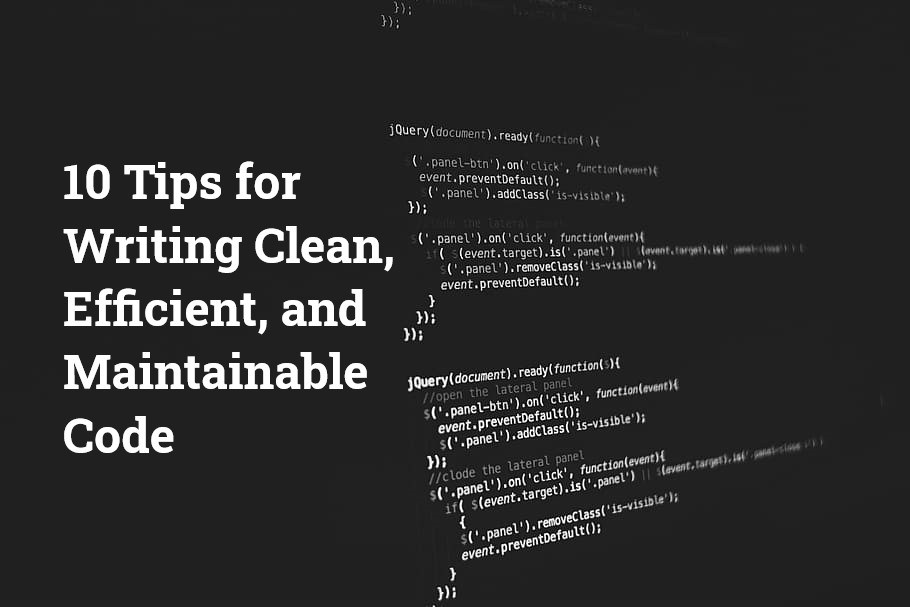
Writing Maintainable and Readable Code
Following Coding Conventions
Writing maintainable and readable code is crucial for the longevity and understanding of any software project. Adhering to coding conventions ensures consistency across the codebase, making it easier for developers to collaborate and comprehend each other’s work.
Here are some essential coding conventions to keep in mind:
- Naming Conventions: Use meaningful variable and function names that accurately reflect their purpose. For example, favor
calculateArea()
over justcalc()
. - Indentation and Spacing: Consistent use of indentation and whitespace enhances readability. It allows developers to quickly understand code structure.
- Commenting Style: Keep comments concise but informative. Use them to explain the “why” behind complex logic rather than the “what,” which should be clear from the code itself.
I remember a challenging debugging session where standardized naming conventions made it much easier to trace issues in our system.
Documenting Code Effectively
Effective documentation complements coding conventions by providing clarity on the functionality and use of code components.
Some best practices include:
- Inline Documentation: Use comments judiciously within the code to explain intricate portions or algorithms, serving as a guide for future developers.
- External Documentation: Maintain comprehensive README files or wikis that outline project setup, usage, and nuances. This is invaluable when onboarding new team members or revisiting old projects.
By prioritizing maintainability and readability through coding conventions and documentation, developers can ensure that their C++ applications are robust and easy to work with over time. Investing in these practices pays off when the codebase scales or changes hands within a team.
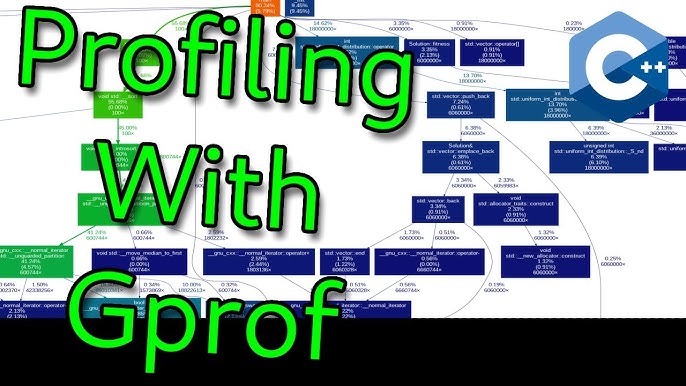
Testing and Profiling for Performance
Importance of Testing
Testing is a critical step in developing high-performance C++ applications, ensuring that code runs effectively and meets specified requirements. It helps identify bugs, performance bottlenecks, and inefficiencies before deployment.
Key reasons why testing is essential include:
- Early Detection of Issues: Testing enables developers to catch potential problems early, reducing the time and cost associated with fixing bugs later in the development cycle.
- Performance Assurance: With rigorous testing, developers can confirm that their optimizations yield the desired performance improvements — ensuring the application operates smoothly under various scenarios.
I recall facing a significant performance issue in a gaming application that could only be pinpointed through rigorous unit and integration testing, leading to a timely resolution.
Profiling Tools and Techniques for C++ Code
Profiling is vital for understanding where time and resources are being spent within your application, allowing developers to make informed optimizations. Several profiling tools and techniques can enhance C++ code performance:
- gprof: A widely-used profiling tool that provides CPU time usage statistics for different functions in a program, helping to identify bottlenecks.
- Valgrind: This tool is excellent for detecting memory leaks and analyzing memory usage, ensuring efficient memory management.
Useful techniques include:
- Sampling: This method helps gather performance data at regular intervals, providing insights into CPU usage over time.
- Tracing: By logging function calls and timings, developers can visualize the workflow and spot inefficiencies.
By integrating effective testing and profiling practices, developers can ensure their C++ applications not only perform well but are also robust and reliable, enhancing the overall user experience.
Case Studies and Examples
Real-World Examples of Efficient C++ Code
To truly grasp the impact of writing efficient C++ code, examining real-world examples can be eye-opening. For instance, consider a high-frequency trading application where milliseconds translate into significant financial losses. In such systems, leveraging multithreading and async I/O operations is crucial. A well-optimized trading engine can process transactions using thread pools, minimizing latency significantly.
Some best practices observed:
- Using Efficient Data Structures: Containers like
std::deque
help manage a dynamic list of trades, optimizing insertion and deletion times. - Incorporating SIMD: For mathematical operations, utilizing Single Instruction, Multiple Data (SIMD) helped process multiple data points simultaneously, enhancing speed.
Performance Comparisons with Inefficient Code
Now, let’s contrast this with inefficient implementations. Imagine a naive trading application that relies on sequential processing and frequently allocates memory during trading loops.
Some pitfalls to avoid:
- Excessive Function Calls: Using non-inlined functions for small, frequently-called operations increased overhead.
- Lack of Memory Pooling: Constant allocation and deallocation led to memory fragmentation and slower performance.
In a side-by-side performance test, the efficient design outperformed the inefficient one by over 30%, highlighting the importance of thoughtful coding practices. By studying these case studies, developers can better appreciate the immense benefits of writing efficient C++ code in real-world applications.