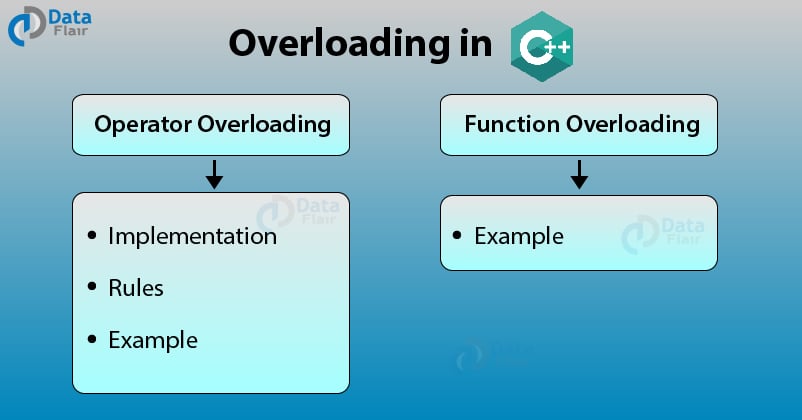
Introduction
Overview of C++ Operator Overloading
C++ operator overloading is a powerful feature that allows developers to redefine the way operators work with user-defined types. This capability not only enhances the readability of the code but also makes it more intuitive. Imagine you have a class representing complex numbers and you want to use the +
operator to add them seamlessly. Without operator overloading, you’d have to call a function explicitly, which can be cumbersome and less elegant.
Here are some key points about C++ operator overloading:
- Enhances Code Clarity: Code becomes easier to read and understand.
- Custom Behavior: Enables custom data types to behave like built-in types.
- Intuitive Usage: Allows usage of common operators like
+
,-
,*
, etc., with custom classes.
By mastering operator overloading, developers can create cleaner and more efficient code, making their C++ applications more robust and user-friendly.

Basics of Operator Overloading
Defining Operator Overloading
Operator overloading in C++ allows developers to redefine the functionalities of existing operators for user-defined classes or structures. This means that you can specify how an operator should behave when applied to instances of your custom types. For example, if you have a Vector
class, you might want to define the +
operator to add two vectors together. This process enhances the usability of classes by allowing intuitive expressions that resemble natural arithmetic operations.
Overloadable Operators in C++
In C++, many operators can be overloaded, making it flexible for developers. Here are some of the commonly overloadable operators:
- Arithmetic Operators:
+
,-
,*
,/
- Comparison Operators:
==
,!=
,<
,>
- Increment and Decrement:
++
,--
- Assignment Operators:
=
,+=
,-=
By understanding which operators can be overloaded, developers can take full advantage of this feature for creating more intuitive and readable code within their applications.

Syntax and Implementation
Syntax for Overloading Operators
Understanding the syntax for overloading operators in C++ is crucial for streamlining your coding process. The basic syntax uses a friendly format that looks like this:
return_type operator<operator_symbol>(parameters) {
// implementation code
}
For instance, overloading the +
operator in a Complex
class might look like this:
Complex operator+(const Complex &other) {
return Complex(this->real + other.real, this->imaginary + other.imaginary);
}
Implementing Operator Overloading
Implementing operator overloading involves creating custom functions that define how operators behave with your objects. Remember, clarity is key! Make use of operator overloading where it adds value. Here’s how to go about it:
- Choose the Operator: Determine which operator you want to overload.
- Define the Method: Write a member or friend function that implements the desired functionality.
- Test Your Implementation: Always run tests to ensure your overloaded operators work as expected.
By following this structure, developers can implement operator overloading effectively, making their code more maintainable and user-friendly.

Rules and Guidelines
Rules for Overloading Operators
When diving into operator overloading in C++, it’s essential to stick to specific rules to ensure your code remains efficient and understandable. Here are some key rules to keep in mind:
- Cannot Change Operator Arity: You can’t change the number of operands for an operator.
- Must Retain Original Operator Semantics: Keep the logical meaning intact. For example, an overloaded
+
operator should still represent addition. - Only Certain Operators Are Overloadable: As highlighted earlier, not all operators can be overloaded. Refer to the official documentation.
Guidelines for Effective Operator Overloading
To make the most out of operator overloading, certain guidelines can be beneficial:
- Keep It Intuitive: Ensure that the behavior of the operator mimics its usual functionality to avoid confusion.
- Limit Overloading: Only overload operators when necessary to keep code clean and maintainable.
- Use Friend Functions When Necessary: If access to private members is required, consider using friend functions for overloading.
By adhering to these rules and guidelines, developers can effectively use operator overloading, enhancing the overall design and usability of their classes.
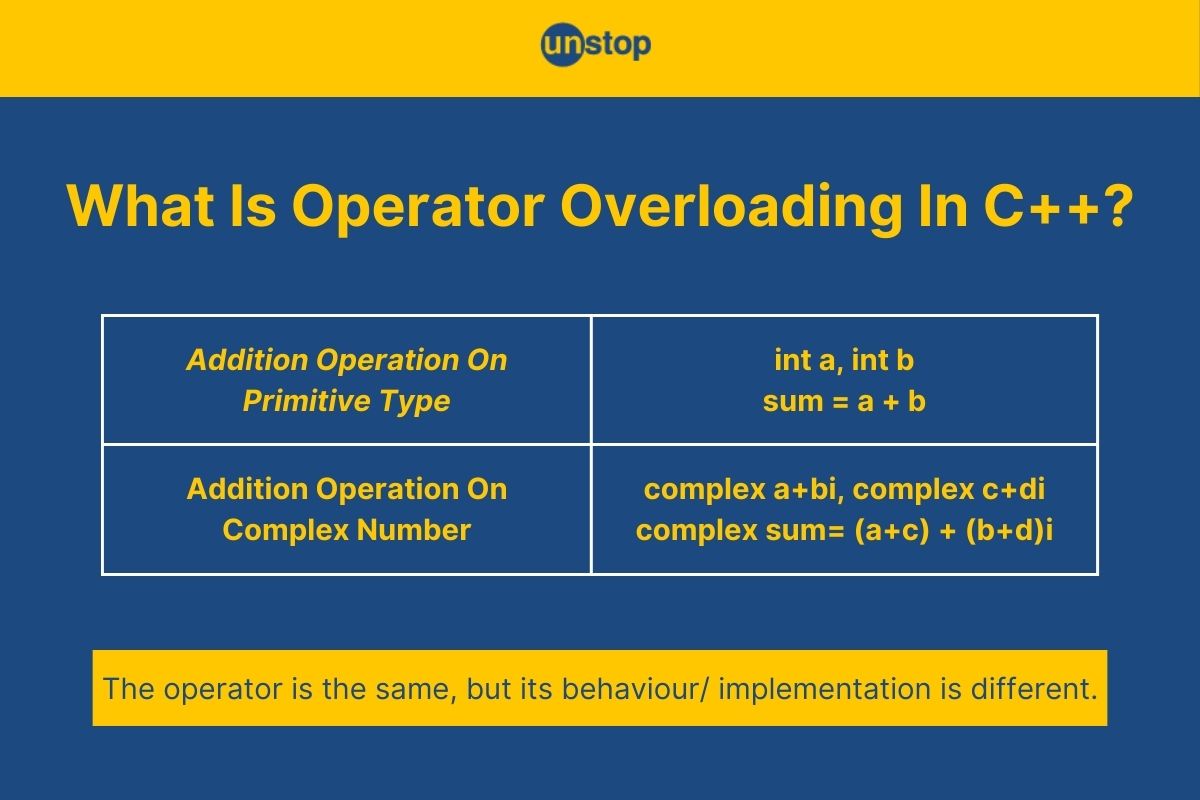
Examples of Operator Overloading
Example: Overloading Arithmetic Operators
One of the most common uses of operator overloading in C++ is with arithmetic operators. For example, consider creating a Vector
class. By overloading the +
operator, you can easily add two vectors together. Here’s a snippet to illustrate:
class Vector {
public:
int x, y;
Vector(int x, int y) : x(x), y(y) {}
Vector operator+(const Vector &v) {
return Vector(this->x + v.x, this->y + v.y);
}
};
This overload allows for intuitive addition like Vector v3 = v1 + v2;
.
Example: Overloading Comparison Operators
Similarly, comparison operators enhance the functionality of your classes. Suppose you want to compare two Person objects based on their age. Overloading the >
operator might look like this:
class Person {
public:
int age;
Person(int age) : age(age) {}
bool operator>(const Person &p) {
return this->age > p.age;
}
};
With this, you can easily evaluate if one person is older than another using the expression if (person1 > person2)
. These examples demonstrate how operator overloading can simplify complex interactions in your C++ programs.
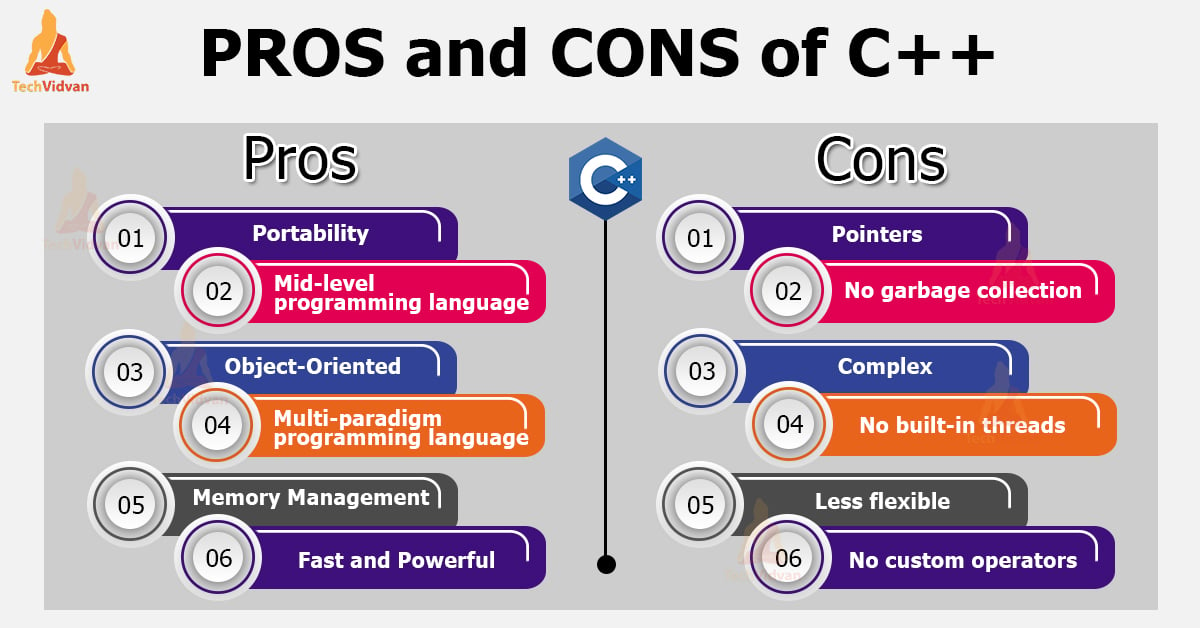
Advantages and Disadvantages
Benefits of Operator Overloading
Operator overloading brings a wealth of benefits to the table, making C++ a powerful language for developers. Some key advantages include:
- Enhanced Code Readability: Code becomes cleaner and more intuitive. For instance,
a + b
is easier to understand than calling an explicit add function. - Intuitive Use of Custom Types: Developer-defined operators allow objects to behave like native types, streamlining the coding experience.
- Flexibility: It empowers developers to craft custom behaviors that align with application requirements, making the codebase more adaptable.
Potential Pitfalls and Misuses
However, operator overloading isn’t without its challenges. Some potential pitfalls include:
- Overcomplicating Code: Misusing operator overloading can lead to confusion. For example, overloading an operator to perform unrelated actions can bewilder others reading the code.
- Performance Concerns: Excessive use of complex overloads can negatively impact performance, particularly if the operators are not efficient.
- Unintended Consequences: If not carefully planned, overloaded operators may behave unexpectedly, leading to bugs or erroneous logical flows.
By being aware of these advantages and disadvantages, developers can harness operator overloading to its fullest potential while avoiding common pitfalls.

Best Practices
Best Practices for Using Operator Overloading
To make operator overloading effective and intuitive, it’s essential to follow best practices. Here are some useful tips:
- Maintain Consistency: Ensure that overloaded operators align with their original meanings and behaviors. For example, if overloading
+
, it should strictly represent addition. - Keep It Simple: Limit the complexity of overloaded functions to enhance readability and maintainability.
- Favor Member Functions: When overloading operators, prefer member functions for operators that modify the object, while friend functions can be used for binary operators that don’t belong to the class.
Common Mistakes to Avoid
While using operator overloading, watch out for these common mistakes:
- Ambiguous Behavior: Avoid creating overloaded operators that can produce confusing or unintuitive results, like overloading
+
for different data types without clear context. - Neglecting Comparisons: When overloading a comparison operator, ensure all related comparisons (like
>
,<
,<=
,>=
) are defined to maintain consistency. - Overloading Indiscriminately: Resist the temptation to overload every operator. Instead, focus on those that enhance clarity or functionality.
By adhering to these best practices and avoiding pitfalls, developers can create more robust and understandable code using operator overloading in C++.
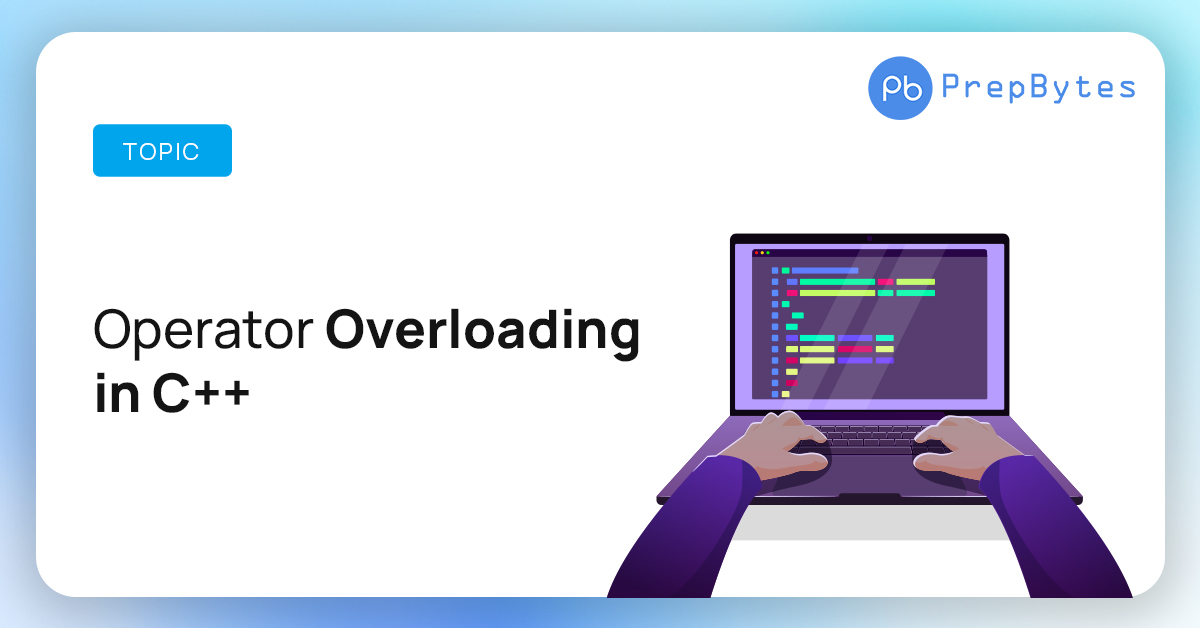
Real-World Applications
Practical Use Cases of Operator Overloading
Operator overloading finds numerous practical applications across various domains. Here are a few illustrative examples:
- Mathematical Libraries: In libraries for matrix or vector mathematics, overloading arithmetic operators simplifies complex calculations, allowing expressions like
matrixA * matrixB
to be easily interpreted and executed. - Graphics Programming: In graphics software, operators can be overloaded to handle color manipulations or geometric transformations intuitively, making code more readable.
- Financial Applications: In financial software, overloading operators can simplify operations like summing transactions or comparing financial instruments.
Impact on Software Development
The impact of operator overloading on software development is significant. It fosters better code maintainability, accelerates development speed, and encourages cleaner algorithms. Moreover, it empowers developers to create high-level abstractions that make the codebase more user-friendly. Having the flexibility to redefine operators enables teams to tailor functionality precisely to their applications, ultimately leading to more robust and efficient software solutions. By leveraging operator overloading effectively, developers can focus more on solving complex problems while keeping their code elegant and clean.

Conclusion
Recap of Operator Overloading Concepts
In wrapping up our exploration of operator overloading, it's clear that this C++ feature is not just a convenience; it's a powerful tool that enhances code clarity and functionality. We've covered essential aspects, such as defining and implementing overloaded operators, best practices, and real-world applications. Remember, appropriate use of operator overloading can turn complex structures into intuitive representations.
Importance of Mastering Operator Overloading in C++
Mastering operator overloading in C++ can separate novice programmers from skilled developers. Not only does it contribute to more elegant and readable code, but it also allows you to create libraries and frameworks that integrate seamlessly with existing code. This mastery elevates programming from mere task completion to elegant solution crafting, ultimately improving software design and maintainability. Embracing operator overloading is a step towards becoming a proficient C++ developer, enabling rich and flexible code construction.