
Overview of C++ Programming
What is C++ Programming?
C++ programming is a powerful, versatile language that extends the C programming language by adding object-oriented features. It’s widely used for developing software applications, games, and system software. C++ is known for its performance and efficiency, making it an ideal choice for high-performance applications.
History of C++ Language
C++ was created by Bjarne Stroustrup in the early 1980s as an enhancement to the C language. Stroustrup wanted to incorporate features such as classes and objects while maintaining the efficiency of C. Over the decades, C++ has evolved through several standards, from C++98 to the latest iterations, introducing new functionalities and improving usability.
Importance of Learning C++ Basics
Learning the basics of C++ is crucial for several reasons:
- Foundation for Other Languages: Many languages like Java and C# draw from C++ principles.
- Understanding Low-Level Programming: C++ provides insights into memory management and system-level programming.
- Career Opportunities: Proficiency in C++ opens doors to roles in game development, robotics, and more.
As you embark on your learning journey, remember that C++ will not only enhance your programming skills but also offer robust career prospects.

Setting Up C++ Environment
Installing a C++ Compiler
To start programming in C++, the first step is to install a C++ compiler. A compiler converts your C++ code into executable programs. Popular choices include:
- GCC (GNU Compiler Collection): Great for Linux users.
- MinGW: A straightforward option for Windows.
- Clang: Known for its excellent diagnostic capabilities.
Installing any of these compilers is typically straightforward; just follow the installation instructions on their official websites.
Choosing an Integrated Development Environment (IDE)
Once you have your compiler, it’s time to select an IDE, which provides a user-friendly interface for writing and managing your code. Consider these top contenders:
- Visual Studio: A robust tool with many features for Windows users.
- Code::Blocks: Lightweight and open-source, perfect for beginners.
- Eclipse CDT: Great for those who prefer a more customizable environment.
Choosing an IDE that fits your style will enhance your coding experience significantly. As you dive into programming with C++, remember that a well-set-up environment lays the groundwork for your learning journey!
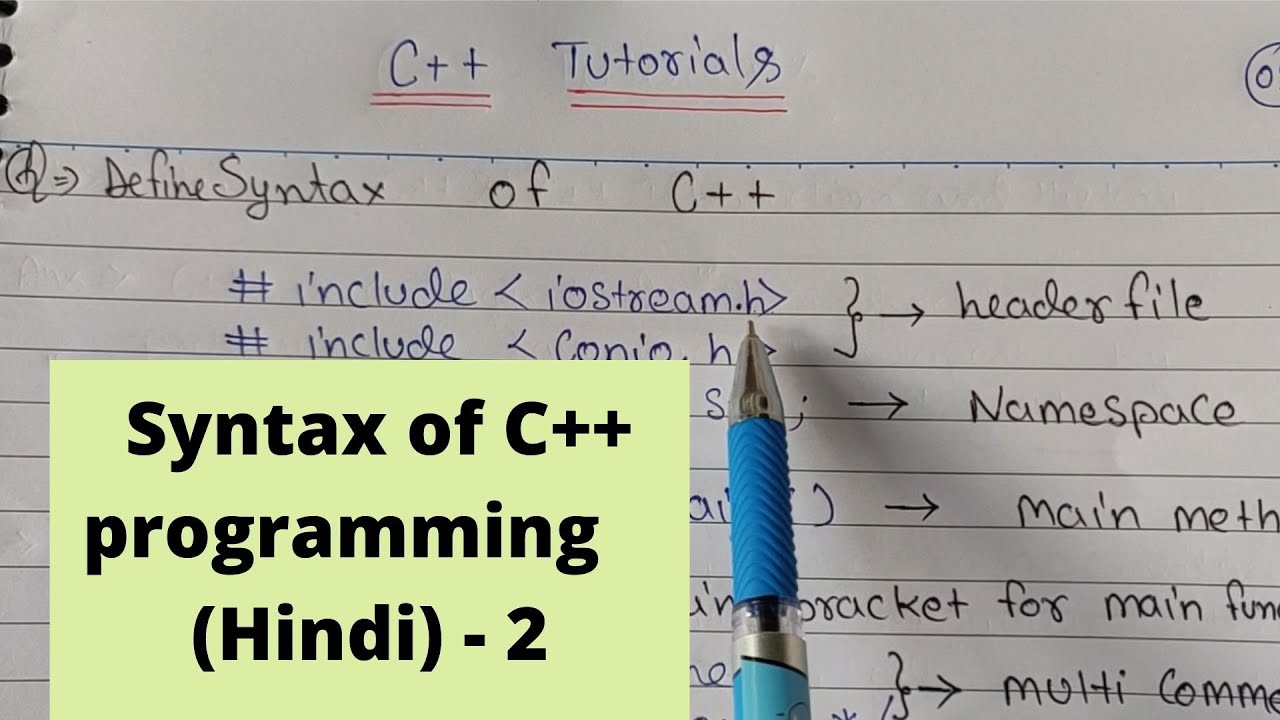
Basic Syntax and Structure
Hello World Program
Now that your environment is set up, let’s create your first C++ program: the classic “Hello, World!” This simple program demonstrates the basic syntax of C++. Here’s how it looks:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
Running this code will display “Hello, World!” in the console, marking the start of your coding adventure!
Variables and Data Types
Understanding variables and data types is crucial. Variables are used to store data, while data types define the type of data stored. Common data types include:
- int: for integers
- float: for floating-point numbers
- char: for characters
- string: for strings of text
Example of declaring a variable:
int age = 25;
float salary = 50000.50;
char initial = 'A';
Operators in C++
Operators allow you to manipulate variables and values. C++ supports various operators, including:
- Arithmetic Operators: +, -, *, /, %
- Relational Operators: ==, !=, <, >, <=, >=
- Logical Operators: && (AND), || (OR), ! (NOT)
Imagine using these operators in your calculations or conditions; they’re the building blocks for creating dynamic programs! With these fundamentals, you’re well on your way to writing more complex C++ programs.
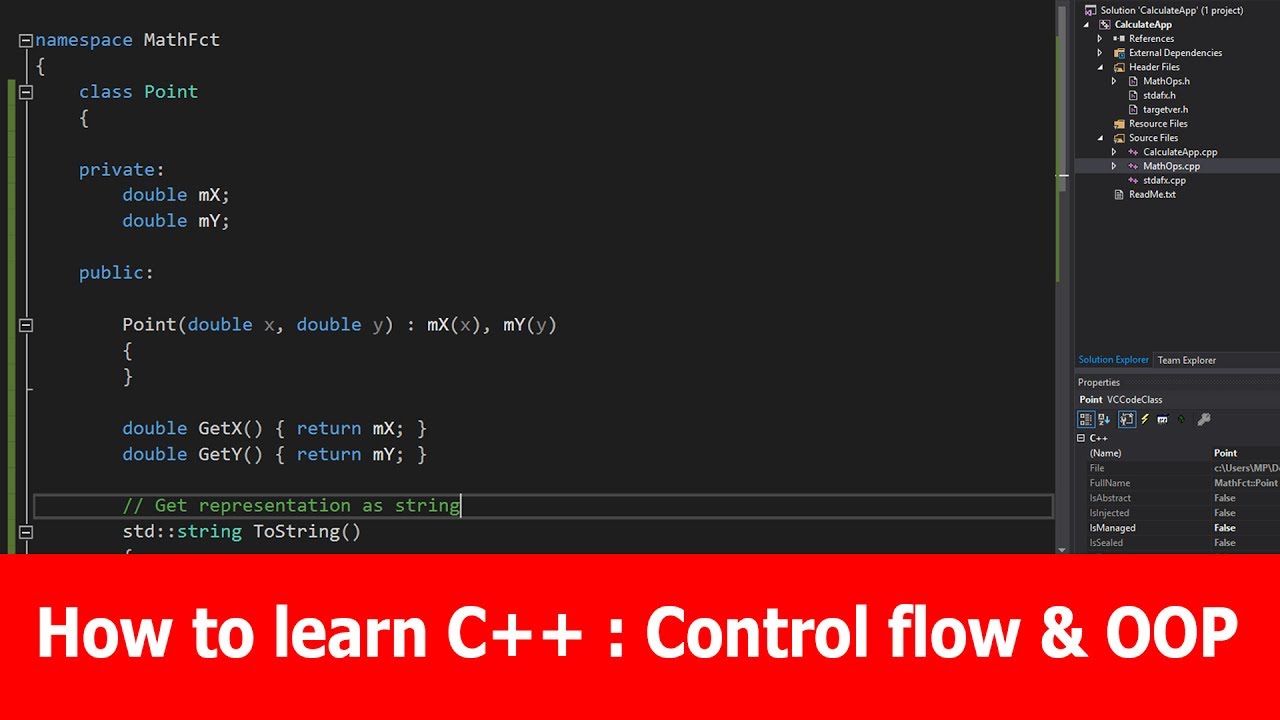
Control Flow in C++
Decision Making with If-Else Statements
Control flow allows your program to make decisions based on conditions. The if-else statement is fundamental for this. Here’s a simple example:
int age = 20;
if (age >= 18) {
cout << "You are an adult!" << endl;
} else {
cout << "You are a minor!" << endl;
}
This structure helps guide your program’s behavior based on user input or other conditions.
Looping with For and While Loops
Now, let’s talk about loops that help repeat tasks. The for loop is great for a known number of iterations. For example:
for (int i = 0; i < 5; i++) {
cout << "Counting: " << i << endl;
}
In contrast, a while loop continues until a condition is false:
int count = 0;
while (count < 5) {
cout << "Count is: " << count << endl;
count++;
}
These loops are invaluable for tasks like iterating through arrays.
Switch-Case Statements
For clearer decision-making with multiple possible outcomes, consider the switch-case statement. Here’s how it works:
int choice = 2;
switch (choice) {
case 1:
cout << "You selected option 1." << endl;
break;
case 2:
cout << "You selected option 2." << endl;
break;
default:
cout << "Invalid choice!" << endl;
}
Switch-case statements can make your code more organized, especially when dealing with multiple conditions. These control flow tools are essential for creating dynamic and responsive C++ programs!
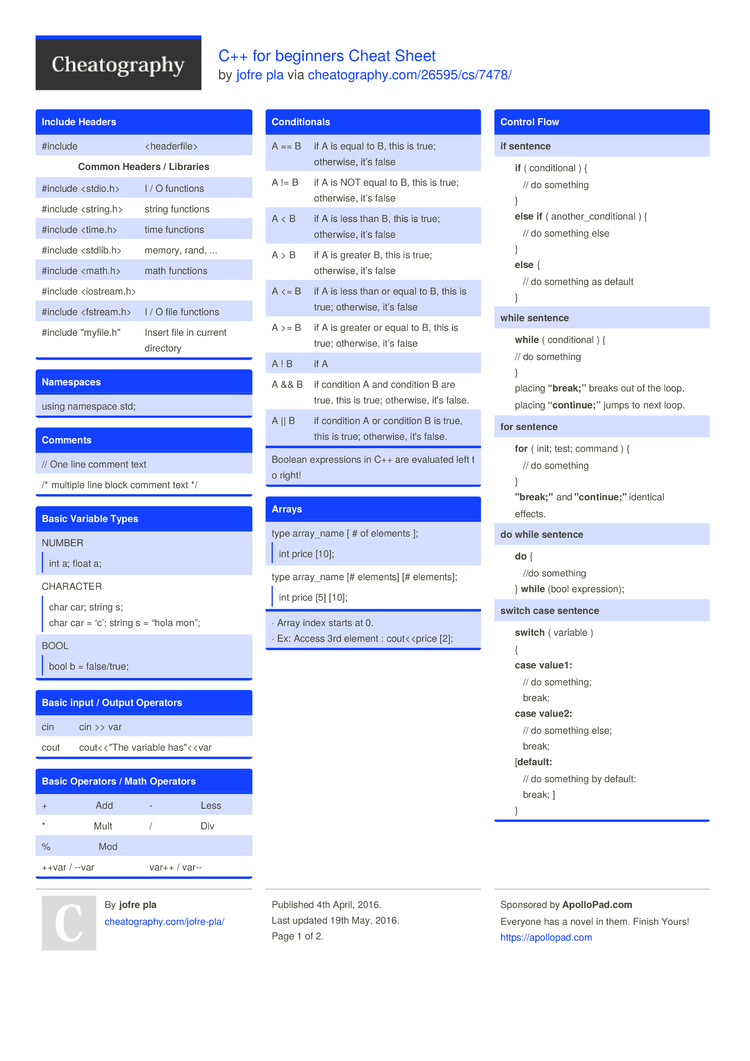
Functions in C++
Creating and Calling Functions
Functions are essential in C++ for organizing your code into reusable blocks. Let’s start by creating a simple function:
void greet() {
cout << "Hello, welcome to C++ programming!" << endl;
}
You can call this function within your main
function like this:
int main() {
greet(); // Function call
return 0;
}
This minimizes code repetition and enhances readability.
Function Parameters and Return Types
Functions can accept parameters, allowing you to pass values to them. Here’s an example:
int add(int a, int b) {
return a + b; // Returns sum of a and b
}
You can call the function with arguments:
int result = add(5, 3);
cout << "Sum: " << result << endl; // Output: Sum: 8
The return type specifies the type of value the function will return, which is useful for further calculations.
Function Overloading
C++ allows function overloading, enabling multiple functions with the same name but different parameters. For example:
int multiply(int a, int b) {
return a * b; // For integers
}
double multiply(double a, double b) {
return a * b; // For doubles
}
This feature enhances code clarity and reduces naming confusion. By mastering functions, you’ll find your coding practices more organized and efficient, paving the way for more complex programs!

Arrays and Pointers
Working with Arrays
Arrays are crucial for storing collections of data in C++. They allow you to manage multiple values of the same type efficiently. Here’s how to declare and initialize an array:
int numbers[5] = {10, 20, 30, 40, 50};
You can access array elements using their index. For instance, numbers[0]
gives you the first element, which is 10.
Understanding Pointers
Pointers are a powerful feature in C++, representing the memory address of a variable. They enable dynamic memory management and efficient data manipulation. Here’s a straightforward example of defining a pointer:
int num = 42;
int* ptr = # // ptr holds the address of num
When you use *ptr
, it dereferences the pointer, giving you the value stored at that address.
Pointer Arithmetic
Pointer arithmetic allows you to perform calculations using pointers, enabling easy navigation through arrays. For example:
int* ptr = numbers; // Points to the first element of the array
cout << *(ptr + 1); // Outputs 20 (the second element)
This is incredibly useful for traversing arrays without using explicit indices. By mastering arrays and pointers, you not only enhance data handling capabilities but also unlock a deeper understanding of memory management in C++.
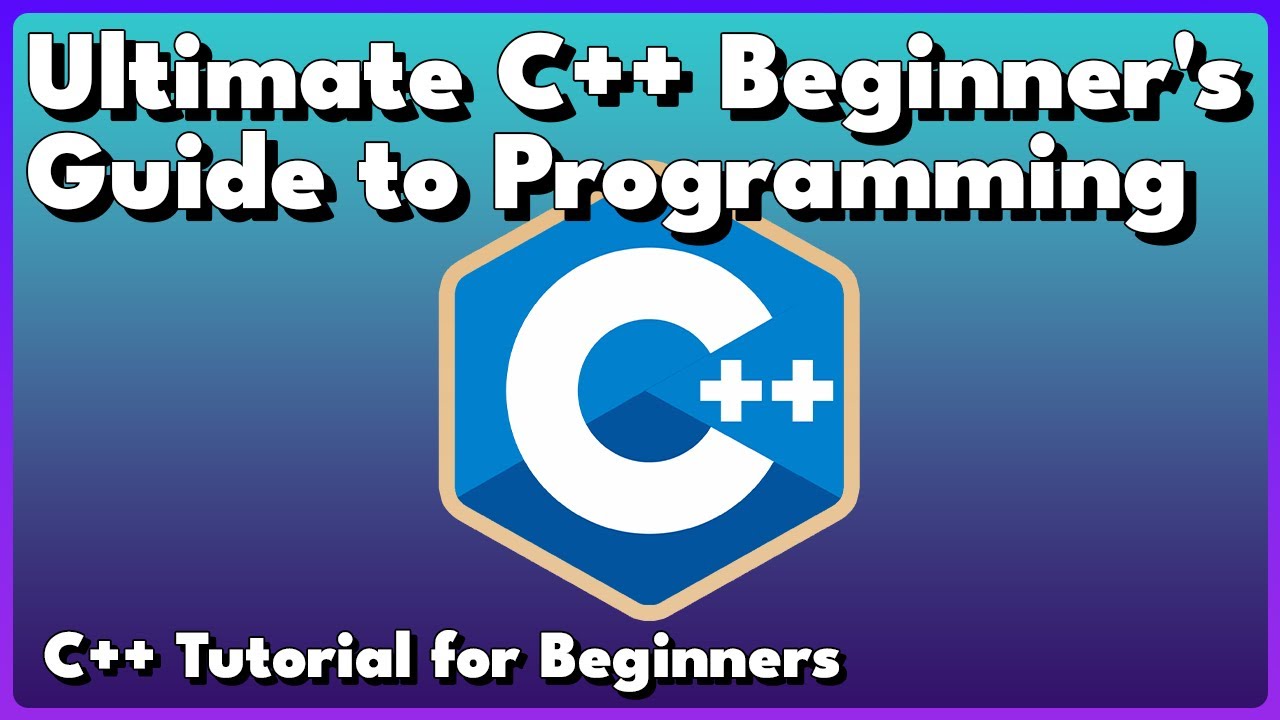
Object-Oriented Programming Concepts
Introduction to Classes and Objects
Object-oriented programming (OOP) is a cornerstone of C++, enabling you to design programs using classes and objects. A class is like a blueprint, while an object is an instance of that blueprint. For example, consider a simple class definition:
class Car {
public:
string model;
int year;
void display() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
You can create an object of the class and invoke its methods:
Car myCar;
myCar.model = "Toyota";
myCar.year = 2020;
myCar.display(); // Outputs: Model: Toyota, Year: 2020
Encapsulation, Inheritance, Polymorphism
Three main concepts define OOP: encapsulation, inheritance, and polymorphism.
- Encapsulation involves bundling data and methods within a class, protecting the internal state.
- Inheritance allows a new class (derived class) to inherit properties and methods from an existing class (base class).
- Polymorphism enables functions to use objects of different classes, enhancing flexibility.
For instance, you could create a SportsCar
class that inherits from Car
.
Constructors and Destructors
Constructors and destructors are special functions in classes. A constructor initializes objects when they are created, while a destructor cleans up resources when objects are destroyed. Here’s a simple example:
class Car {
public:
Car() { // Constructor
cout << "Car object created." << endl;
}
~Car() { // Destructor
cout << "Car object destroyed." << endl;
}
};
With constructors and destructors, you ensure that your application manages memory effectively. Embracing these OOP concepts will empower you to write organized, maintainable, and scalable C++ programs!

Memory Management
Stack vs. Heap Memory
Understanding memory management is essential in C++. There are two primary types of memory: stack and heap.
- Stack Memory: This is used for static memory allocation. It holds local variables and is automatically managed. When a function exits, its stack memory is released.
- Heap Memory: This is used for dynamic memory allocation, allowing you to allocate and deallocate memory manually using pointers.
For example, if you create an array of unknown size during runtime, you’d use heap memory.
Dynamic Memory Allocation with new and delete
In C++, you can manage heap memory using the new
and delete
operators. Here’s how it works:
int* arr = new int[5]; // Dynamic array allocation on the heap
// use the array...
delete[] arr; // Freeing the allocated memory
Using new
allocates memory on the heap, while delete
releases it. This is crucial for preventing memory issues in larger applications.
Memory Leaks and How to Avoid Them
A memory leak occurs when memory is allocated but not properly deallocated, leading to wasted resources. To avoid memory leaks:
- Always pair
new
withdelete
andnew[]
withdelete[]
. - Use smart pointers (e.g.,
std::unique_ptr
,std::shared_ptr
) from the C++ Standard Library, which automatically manage memory. - Regularly review your code for any allocated but unfreed memory.
By practicing good memory management, you can ensure your C++ applications run efficiently and effectively, paving the way for more advanced programming!
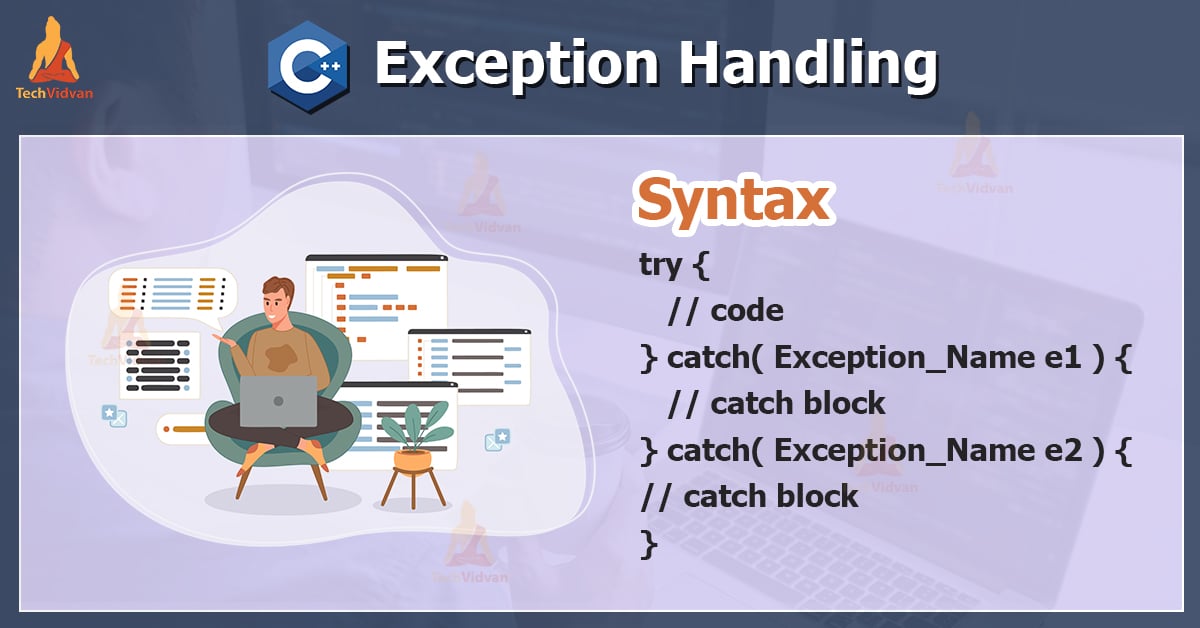
Exception Handling
Handling Errors with Try-Catch Blocks
Exception handling is a crucial aspect of C++ programming, allowing developers to manage errors gracefully. The try-catch block helps you encapsulate code that might throw exceptions. For example:
try {
// Code that might cause an exception
int result = 10 / 0; // Division by zero
} catch (const runtime_error& e) {
cout << "Error: " << e.what() << endl; // Handle the exception
}
In this case, if an error occurs, the catch block will execute, preventing your program from crashing.
Throwing and Catching Exceptions
You can explicitly throw exceptions using the throw
keyword. This is useful for signaling an error condition. For instance:
void checkAge(int age) {
if (age < 0) {
throw runtime_error("Age cannot be negative!");
}
}
When calling this function, you can handle the exception in a try-catch block as demonstrated earlier.
Custom Exception Classes
Sometimes, built-in exceptions aren’t enough, and you may need to define your own custom exception classes. This allows you to provide more context about the error:
class MyException : public exception {
public:
const char* what() const noexcept override {
return "This is a custom exception!";
}
};
// Using the custom exception
try {
throw MyException();
} catch (const MyException& e) {
cout << e.what() << endl;
}
Creating custom exceptions enhances the clarity of your error handling. By mastering exception handling, you unlock the ability to build robust and error-tolerant C++ applications!

Advanced Topics in C++
Templates and Generic Programming
Templates in C++ allow you to write generic functions and classes that can operate with any data type. This is a powerful feature that promotes code reusability and reduces redundancy. For example:
template <typename T>
T add(T a, T b) {
return a + b;
}
You can call this function with various types, like integers or floats, making your code versatile and adaptable.
Standard Template Library (STL)
The Standard Template Library (STL) is a collection of template classes and functions that provide general-purpose data structures and algorithms. Some common components include:
- Vectors: Dynamic arrays that can resize.
- Lists: Doubly linked lists for efficient insertion and deletion.
- Maps: Associative arrays (key-value pairs).
Using STL can significantly simplify your programming tasks, as it provides ready-to-use, efficient implementations of complex data structures, letting you focus on your application’s logic.
Multithreading with C++
As applications become more complex, multithreading becomes essential for improving performance. C++11 introduced a robust threading library, allowing you to create and manage multiple threads easily. Here’s a quick example:
#include <thread>
void printMessage() {
cout << "Hello from thread!" << endl;
}
int main() {
std::thread myThread(printMessage); // Creating a thread
myThread.join(); // Wait for the thread to finish
return 0;
}
Multithreading enhances the efficiency of your applications, especially during resource-intensive tasks. By embracing these advanced topics in C++, you’ll be well-equipped to tackle complex programming challenges and create high-performance applications!
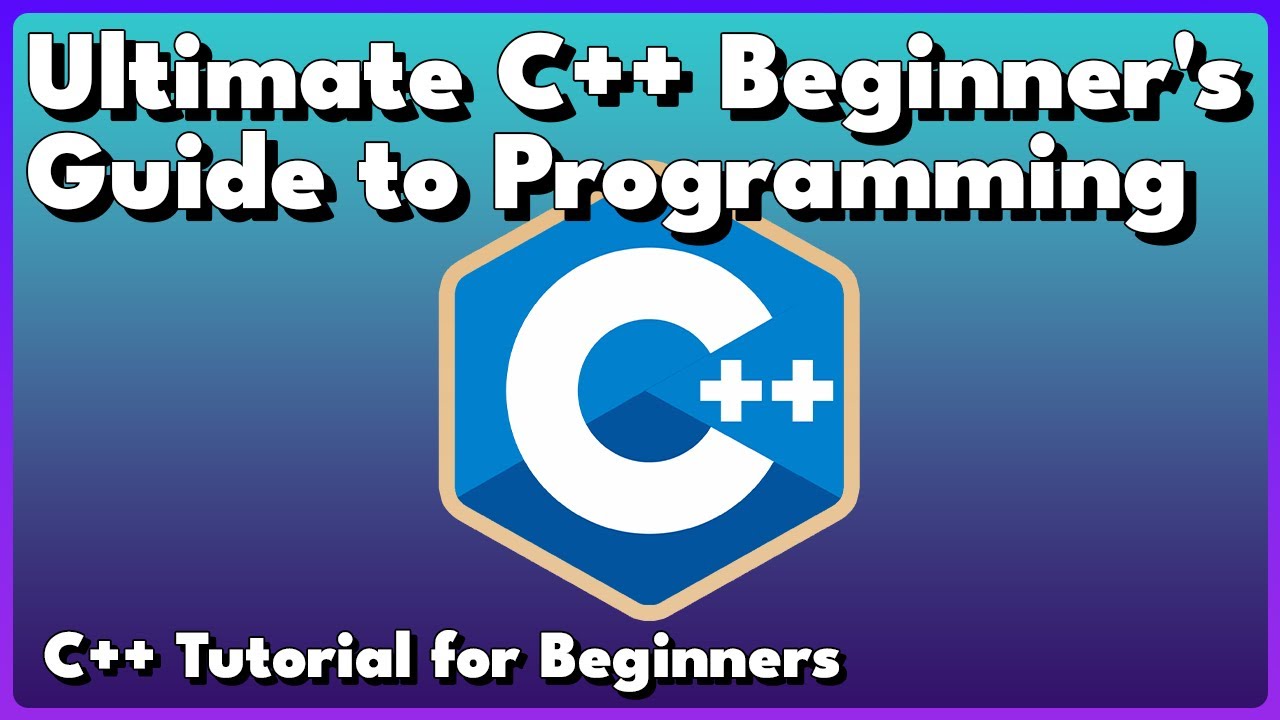
Best Practices and Coding Standards
Naming Conventions
Adhering to consistent naming conventions is crucial for enhancing code clarity. A good practice is to use meaningful names that describe the purpose of variables, functions, and classes. For example:
- Use camelCase for variables (e.g.,
userAge
). - Use PascalCase for class names (e.g.,
EmployeeRecord
). - Keep function names descriptive, like
calculateTotalSalary()
.
This not only makes your code easier to read but also simplifies collaboration with others.
Code Readability and Maintainability
Creating readable code is an art that pays off in the long run. Here are some tips to enhance readability:
- Use consistent indentation and spacing.
- Break code into small, well-defined functions.
- Keep your lines of code concise; aim for clarity over cleverness.
By focusing on maintainability, future developers—and even your future self—will find it easier to understand and modify your code.
Commenting and Documentation
Effective commenting can bridge gaps in understanding. Use comments to explain the “why” behind complex logic rather than the “what,” which should be clear from the code itself. Implementing documentation practices, such as using Doxygen or other tools, can formalize this. For example, document the purpose of classes and functions to provide quick reference points.
Good documentation and comments not only enhance user experience but also serve as a valuable guide for future maintenance. By adopting these best practices, you’ll cultivate a coding style that encourages clarity, collaboration, and longevity in your C++ projects!

Debugging and Troubleshooting
Using Debugging Tools
Debugging is an essential skill for any programmer, and C++ offers several powerful debugging tools. Integrated Development Environments (IDEs) like Visual Studio and Code::Blocks provide built-in debuggers that allow you to set breakpoints, step through code, and inspect variable values. Using tools like GDB (GNU Debugger) is also beneficial for command-line enthusiasts, where you can interactively debug your applications and find issues effectively.
Common C++ Errors and How to Fix Them
As you dive deeper into C++, you might encounter common errors such as:
- Segmentation Faults: Often caused by accessing invalid memory. Check pointers and array indices.
- Compilation Errors: Usually related to syntax issues. Read the error messages closely to identify the line of code in question.
- Linker Errors: Occur when the compiler can’t find the implementation of a declared function. Ensure all included files are linked correctly.
These errors are part of the learning process; don’t let them discourage you!
Tips for Effective Troubleshooting
To troubleshoot effectively, consider these tips:
- Read Error Messages: Often, compiler and runtime messages provide hints about the problem.
- Simplify Your Code: Isolate problematic code by commenting out sections to narrow down the source of issues.
- Keep Learning: Utilize online forums or community support; sometimes a fresh perspective can reveal a solution.
By embracing debugging and troubleshooting, you’ll transform obstacles into opportunities for growth in your C++ programming journey!
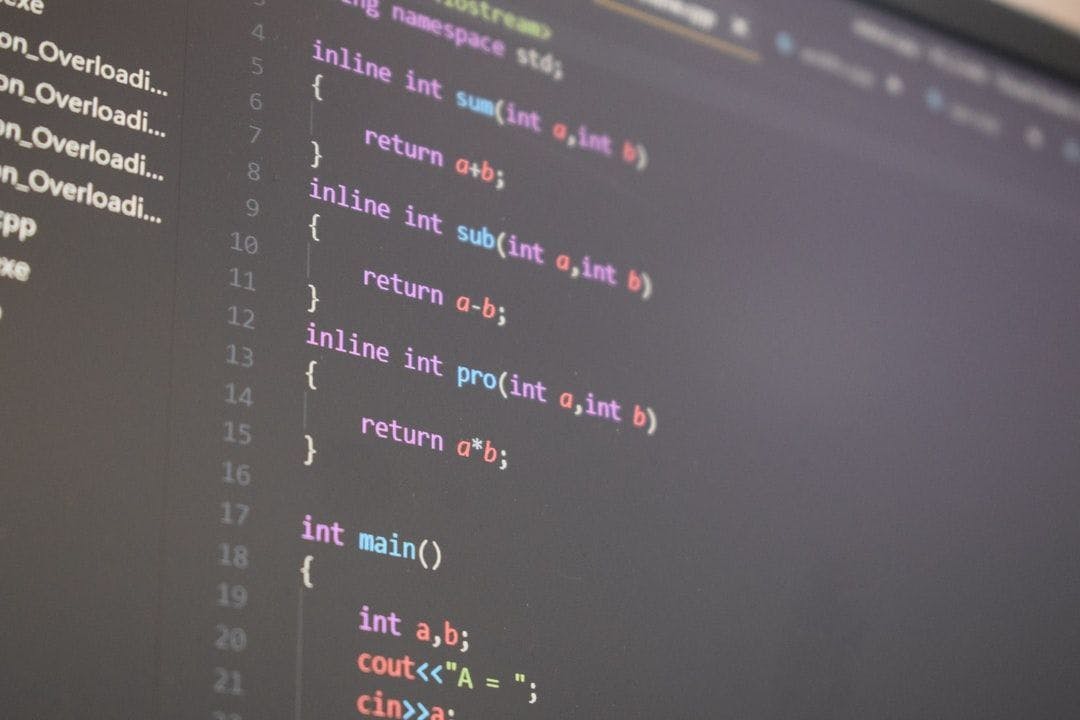
XIII. Conclusion
Summary of Key Learnings
As you wrap up your journey through C++, you’ve covered a wealth of knowledge—from basic syntax and control flow to advanced concepts like object-oriented programming and multithreading. Key takeaways include:
- The importance of understanding memory management and debugging techniques.
- How to implement best practices in coding for clarity and maintainability.
- The versatility provided by templates and the Standard Template Library (STL).
Embracing these principles will enhance your coding proficiency.
Next Steps in Mastering C++ Programming
Now that you’ve built a solid foundation, consider the following next steps:
- Build Projects: Start with small projects and gradually increase complexity to implement what you’ve learned.
- Explore Libraries and Frameworks: Familiarize yourself with frameworks like Qt or Boost to widen your programming capabilities.
- Contribute to Open Source: Joining open source projects can provide real-world experience and community support.
With continuous practice and exploration, you’ll refine your skills and advance in your C++ programming journey. Happy coding!